새로 배운 것 : for문, globals함수
그동안 변수를 임의로 프로그램 내에서 동적으로 만드는 것이 불가능하다고 생각했는데, 이걸 파이썬이 가능할 줄은 몰랐다. 다만 전역변수를 선언하는 방식이라 권장하진 않는다. 삭제도 될까 했는데 가능하지만, 속도면에서 좋을지는 아직 잘 모르겠다. 오늘도 새로운 걸 배워서 좋았다.
for i in range(1, 10):
globals()[f'a_{i}'] = i + 3
print(a_1)
print(a_2)
print(a_3)
print(a_4)
print(a_5)
# 파이썬은 글로벌로 변수명 선언이 가능하다.
# 다만 글로벌 변수가 늘어나기 때문에 권장하지 않는 방법임
# 그럼 지우는건 될까?
for i in range(1, 6):
globals().pop(f'a_{i}')
# 가능하다!
# print(a_1) # error !
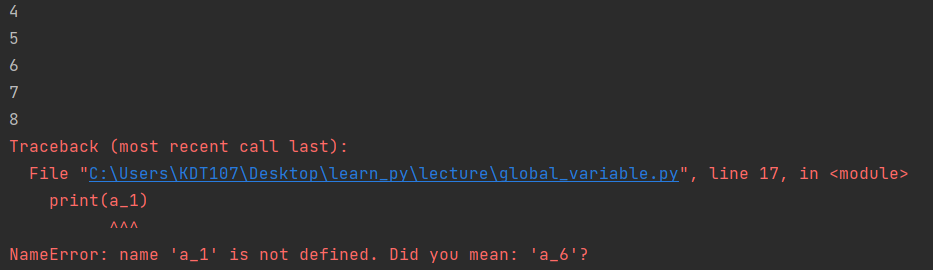
이전에 Java의 Spring을 공부하면서 메시징, 국제화 방법에 대해서 공부한 적이 있다. 바로, 화면이나 웹에 출력할 공통 메세지들을 하나의 파일에서 관리한다. 메시지는 메시지대로, 로직은 조회만 하고(추후엔 이것을 '의존한다' 라고 함), 로직에만 집중하는 Single Responsibility Principle을 준수하려는 전략이다. (만들어 놓으면 편한 것이지, 만드는게 편하다는 뜻은 아니다)
이 방법을 활용해 print로 매 분기마다 직접 타이핑한 것을 출력하는 것 대신, 설계에서 미리 text 를 관리하도록 해본다. 오늘의 예제는 가위바위보 게임 만들기이다.
import random
import os
# TODO: 변수 선언
computer_select_num = None
user_select_num = None
intro_message = ["가위바위보 게임입니다. 무엇을 내시겠습니까?\n", '[ 1. 가위 ] [ 2. 바위 ] [ 3. 보 ]\n', '번호를 선택하세요. : ']
select_message = ['[ 가위 ]', '[ 바위 ]', '[ 보 ]', '를 입력하셨습니다.\n']
verified_message = ['잘못된 입력 입니다. 다시 입력 해주세요.\n']
verified_domain = ['1', '2', '3']
result_message = ['승리하였습니다.\n', '비겼습니다.\n', '패배하였습니다.\n', '컴퓨터가 낸 것은', '[ 가위 ]였습니다.\n', '[ 바위 ]이였습니다.\n', '[ 보 ]였습니다.\n']
statistic_message = ['총 전적은 ', '판입니다.', '승리 횟수 : ', '비긴 횟수 : ', '패배 횟수 : ', '승률 : ']
retry_ask_message = ['한번 더 하시겠습니까?\n', '[ 1. 계속 ] [ 2. 그만 ]\n', '번호를 선택하세요. : ']
exit_message = ['정상적으로 종료되었습니다.']
is_program_going = True
game_statistic = {
'total_play_count': 0,
'win_count': 0,
'draw_count': 0,
'lose_count': 0,
}
while is_program_going:
# TODO: 컴퓨터가 뽑은 수
computer_select_num = random.randrange(1, 3)
game_result = 0 # 게임 결과에 따라 game_statistic 반영 예정
os.system("cls") # 화면 지우기
while True:
# TODO: 유저 입력
for m in intro_message:
print(m, sep='', end='')
user_select_num = input()
# TODO: 유저 입력 검증
if user_select_num not in verified_domain:
print()
for m in verified_message:
print(m, sep='', end='\n')
else:
user_select_num = int(user_select_num)
if user_select_num == 1:
print(select_message[0], end='')
elif user_select_num == 2:
print(select_message[1], end='')
elif user_select_num == 3:
print(select_message[2], end='')
print(select_message[3])
break # 검증 완료
# TODO: 승부 검증
if user_select_num == 1: # 유저 가위 낸 경우
if computer_select_num == 1: # 컴퓨터 가위 낸 경우 : 서로 비김
game_result = 2
elif computer_select_num == 2: # 컴퓨터 바위 낸 경우 : 유저 짐
game_result = 3
elif computer_select_num == 3: # 컴퓨터 보 낸 경우 : 유저 이김
game_result = 1
elif user_select_num == 2: # 유저 바위 낸 경우
if computer_select_num == 1: # 컴퓨터 가위 낸 경우 : 유저 이김
game_result = 1
elif computer_select_num == 2: # 컴퓨터 바위 낸 경우 : 서로 비김
game_result = 2
elif computer_select_num == 3: # 컴퓨터 보 낸 경우 : 유저 짐
game_result = 3
elif user_select_num == 3: # 유저 보 낸 경우
if computer_select_num == 1: # 컴퓨터 가위 낸 경우 : 유저 짐
game_result = 3
elif computer_select_num == 2: # 컴퓨터 바위 낸 경우 : 유저 이김
game_result = 1
elif computer_select_num == 3: # 컴퓨터 보 낸 경우 : 서로 비김
game_result = 2
# TODO: 승부 결과 통계 반영
game_statistic['total_play_count'] += 1
if game_result == 1: # 승리
game_statistic['win_count'] += 1
elif game_result == 2: # 비김
game_statistic['draw_count'] += 1
elif game_result == 3: # 패배
game_statistic['lose_count'] += 1
# TODO: 결과화면 출력
if game_result == 1: # 승리
print(result_message[0], end='')
elif game_result == 2: # 비김
print(result_message[1], end='')
elif game_result == 3: # 패배
print(result_message[2], end='')
print(result_message[3], end=' ')
if computer_select_num == 1:
print(result_message[4])
elif computer_select_num == 2:
print(result_message[5])
elif computer_select_num == 3:
print(select_message[6])
# TODO: 통계화면 출력
print(f"""{statistic_message[0]}{game_statistic['total_play_count']} {statistic_message[1]}
{statistic_message[2]}{game_statistic['win_count']}
{statistic_message[3]}{game_statistic['draw_count']}
{statistic_message[4]}{game_statistic['lose_count']}
{statistic_message[5]}{(game_statistic['win_count'] * 100) / game_statistic['total_play_count']:.2f} %
""")
# TODO: 다시 시작할지 물어보기
while True:
# TODO: 유저 입력
for m in retry_ask_message:
print(m, sep='', end='')
user_select_num = input()
# TODO: 유저 입력 검증
if user_select_num not in verified_domain[:2]: # 1, 2까지만 확인함
print()
for m in verified_message:
print(m, sep='', end='\n')
else:
user_select_num = int(user_select_num)
if user_select_num == 1: # 다시 시작하는 경우
pass
elif user_select_num == 2: # 그만하는 경우
is_program_going = False
break # 검증 완료
# 종료 메시지
for m in exit_message:
print(m, sep='', end='')
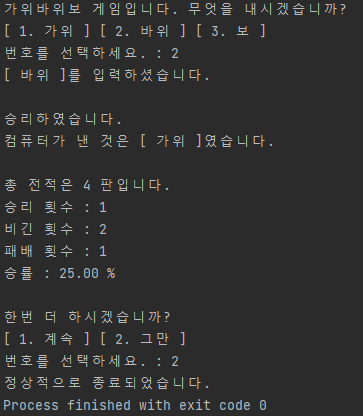
로직 코드를 보면, print에서 직접 문자열을 타이핑하는 것이 하나도 없다.
선택지에 따라 1~3을 물어보거나 1~2를 물어볼 수 있다. if x in range(1,3) 이런식으로 문장을 구성해도 되지만,
verified_domain을 활용하여 추후에 입력을 받는 방법이 달라지더라도 입력 도메인만 변경해주면 그만이다.
반복문까지 배웠으면, 이제 곧 함수와 클래스에 대한 개념을 배울 것이고, 객체지향적인 사고가 슬슬 요구되기 시작할 것이다.
늘 처음엔 힘들고 어렵다. 하지만 포기하면 끝나는 것이다. 익숙해질 때까지 계속 반복 숙달하기.
그것이 익숙해지고 나면 늘 그렇듯 새로운 난관이 기다리고 있을 것이다.
하나하나씩 넘고, 힘들면 좀 쉬고, 다른길도 돌아보고 바람도 쐬고 ...
그리고 결국 자기가 원하는 것하면서 사는게 좋은 패턴인 것 같다.
감사합니다.
'광주인력개발원' 카테고리의 다른 글
<경주마와 아이들> 팀이 내주신 문제 풀이 중간 보고서 <2023-04-24 개발일지> (0) | 2023.05.30 |
---|---|
for 반복문 사용하여 숫자 스무고개 만들기 [2023-04-21] (1) | 2023.05.30 |
<김주김> 팀이 내주신 문제 풀이 완료 [2023-04-20 학습일지] (0) | 2023.05.30 |
<동녘짱과 아이들> 팀이 내주신 문제 풀이 완료 [2023-04-20 학습일지] (0) | 2023.05.30 |
다시 돌아온 별찍기 파이썬 버전 [2023-04-20] (1) | 2023.05.29 |