후기 :
2일동안 엄청 풀어보았다. 그러면서 느낀건. 자료형을 활용하는 것, 코딩하는 방식에 대해 스타일이 발전하게 되었다.
단순 리스트, 딕셔너리만 사용하던 타입에서 필요에 따라
딕셔너리 기본 골격에 인덱스를 쓸 수 있게 정수를 키로 설정해주고, 필요한 자료형을 넣는 방식으로 바꼈다.
0 : [ 리스트 ],
1 : [ 리스트 ] 또는
2 : [ 딕셔너리 ] 방식
이렇게 되면 유저가 입력한 값을 바로 딕셔너리에서 가져올 수가 있게 된다.
또한 딕셔너리를 자주 쓰다보면, 객체지향적 프로그래밍을 할 수 있게 되어, 반쪽짜리 클래스를 쓰는 느낌이 든다.
(함수가 없기 때문에 C의 구조체 느낌)
일단 딕셔너리로 사용하고 있어 소스 코드 자체는 현재 지저분하게 보이지만, 추후에 클래스, 함수 선언, getter 나 setter를 사용하게 되면 이전보다 더 깔끔한 디자인의 소스코드로 직관적이게 되리라 생각한다.
코딩하는 방식은 그동안 #을 이용해 간단히 할 일만 적는 설명으로만 썼는데, <월요일이조>에서 올려준
# TODO : ???
방식을 활용하니 소스코드도 조금더 눈에 띄고 할일이 직관적이라 머리속에서 할일이 정해지고 큰 틀에서부터 작은 소스 부분으로 짜는게 수월해졌다. (할일만 적고 소스코드만 달았는데 주석이 달려서 나오는 소스코드가 됨)
다음으로는 2일간 정리한 소스코드이다.
팀별 업로드 문제와 풀이 방식, 느낀점으로 접근해보겠다.
"""
### 문제1 (팀명: 동녘짱과 아이들)
# 사용자가 입력한 월(정수형)이 28일까지 있는지, 30일까지 있는지, 31일까지 있는지 조건문으로 확인하라
# 1. 입력한 값이 2월이면 28일까지 있음을 전하는 메시지 출력
# 2. 입력한 값이 4, 6, 9, 11월이면 30일까지 있음을 전하는 메시지 출력
# 3. 입력한 값이 1, 3, 5, 7, 8, 10, 12월이면 31일까지 있음을 전하는 메시지 출력
"""
if quest_select == 1:
print('\n문제1 (팀명: 동녘짱과 아이들)')
user_input_month = int(input('[윤년 아닌 기준]몇 번째 달이 궁금합니까?'))
print(f'{user_input_month}을 입력 하셨습니다.')
user_input_month -= 1 # 인덱스 보정
days_of_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if 1 <= user_input_month <= 12:
print(f'{days_of_month[user_input_month]} 일 입니다.')
else:
print('잘못 입력하셨습니다.')
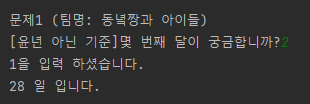
윤년은 계산하지 않았다. 방식은 알지만, 그게 메인은 아닌 것 같아서 단순 조건문과 리스트를 활용하여 풀었다.
대신 사용자가 1~12 내의 숫자가 입력됐는지 검증 기능을 추가했다.
"""
### 문제 2 (팀명: 동녘짱과 아이들)
# 1. 당신의 국어/영어/수학/과학 점수를 입력하고
# 2. 국어/영어/수학/과학의 평균 점수를 구한 후
# 3. 그 평균 점수로 A+부터 F까지 당신이 임의로 구간을 정해서
# 4. 학점을 출력하라
"""
if quest_select == 2:
print('\n문제2 (팀명: 동녘짱과 아이들)')
user_korea_score = int(input('국어 점수가 몇 점입니까?'))
user_english_score = int(input('영어 점수가 몇 점입니까?'))
user_math_score = int(input('수학 점수가 몇 점입니까?'))
user_science_score = int(input('과학 점수가 몇 점입니까?'))
score_avg = (user_korea_score + user_english_score + user_math_score + user_science_score) / 4
grade = 'F'
if 95 <= score_avg:
grade = 'A+'
elif 90 <= score_avg < 95:
grade = 'A'
elif 85 <= score_avg < 90:
grade = 'B+'
elif 80 <= score_avg < 85:
grade = 'B'
elif 75 <= score_avg < 80:
grade = 'C+'
elif 70 <= score_avg < 75:
grade = 'C'
elif 65 <= score_avg < 70:
grade = 'D+'
elif 60 <= score_avg < 65:
grade = 'D'
print(f'평균은 {score_avg:.2f}입니다.')
print(f'당신의 학점은 {grade} 입니다.')
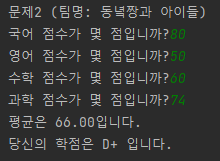
단순 계산 평균 구하는 문제였다. 대신 소수가 길게 나오는게 보기 싫어서 서식 문자를 사용해보았다.
"""
### 문제 3 (팀명: 동녘짱과 아이들)
# menu = [ '고봉민김밥', '라면', '돈까스']
# price = [ 3000, 4000, 9000 ]
# 1. 위의 리스트를 선언
# 2. 위의 리스트를 다시 하나의 딕셔너리로 합친 후, 이를 이용해서 아래의 내용을 구현하시오.
# 3. 사용자가 구매할 메뉴를 입력 받음
# 4. 사용자의 지불 금액을 입력 받음
# 5. 결제금액보다 사용자의 지불금액이 높으면 잔액 및 구매 메세지 출력
# 6. 결제금액보다 사용자의 지불금액이 낮으면 잔액 부족과 돈을 돌려주는 메시지 출력
"""
if quest_select == 3:
print('\n문제3 (팀명: 동녘짱과 아이들)')
menu = ['고봉민김밥', '라면', '돈까스']
price = [3000, 4000, 9000]
menupan = dict()
menupan.update({1: [menu[0], price[0]]})
menupan.update({2: [menu[1], price[1]]})
menupan.update({3: [menu[2], price[2]]})
print(f"""
음식점 메뉴판
1.{menupan[1][0]} {menupan[1][1]}
2.{menupan[2][0]} {menupan[2][1]}
3.{menupan[3][0]} {menupan[3][1]}
""")
menu_selection = int(input('어떤 메뉴를 고르시겠습니까? '))
user_money = int(input('얼마를 지불하시겠습니까? '))
menu_price = 0
if menu_selection == 1:
menu_price = menupan[1][1]
if menu_selection == 2:
menu_price = menupan[2][1]
if menu_selection == 3:
menu_price = menupan[3][1]
if menu_price <= user_money:
print(f'[구매 성공!] 잔액 {user_money - menu_price} 원 돌려드리겠습니다.')
else:
print(f'[구매 실패!] 돈이 부족합니다. {user_money} 원 다시 돌려드리겠습니다.')
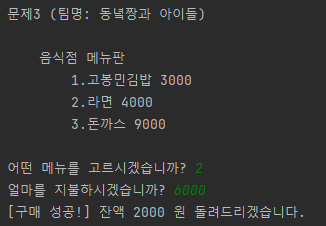
간단한 파이썬 버전의 김밥천국 문제였다. 문제를 월요일에 풀었는데, 수요일인 지금 다시 저 문제를 보았을 때, 자료형을 한 리스트에 이름과 가격을 같이 넣어 딕셔너리에 넣었으면 더 좋지 않았을까 싶다. 전반적으로 교수님이 생각하신 출제의도 내에 풀 수 있게끔, 너무 어렵지도 않게 잘 출제 해주셨다.
"""
팀 : 똘똘이
Q1. 2023년을 기준으로
월, 일을 입력 받아서 공휴일인 아닌지 알려준다.
공휴일이면 "공휴일 입니다." 라고 출력
공휴일이 아니면 "공휴일이 아닙니다. " 라고 출력한다.
좀 더 귀찮은걸 좋아하는 분은 무슨 날인지까지 출력
ex)어린이 날입니다.
"""
if quest_select == 7:
print('\n문제1 (팀명: 똘똘이)')
holiday_list = {
1: [1, 8, 15, 29, 21, 22, 23, 24],
2: [5, 12, 19, 26],
3: [1, 5, 12, 19, 26],
4: [2, 9, 16, 23, 30],
5: [5, 27, 7, 14, 21, 28],
6: [6, 4, 11, 18, 25],
7: [2, 9, 16, 23, 30],
8: [15, 6, 13, 20, 27],
9: [28, 29, 30, 3, 10, 17, 24],
10: [3, 9, 1, 8, 15, 22, 29],
11: [5, 12, 19, 26],
12: [25, 3, 10, 17, 24, 31],
}
result = ''
result_detail = ''
user_input = input('공휴일인지 궁금한 날짜를 입력하세요. 예시) 3/4 -> 3월 4일로 해석됨\n')
month, day = user_input.split('/')
month = int(month)
day = int(day)
if day in holiday_list.get(month):
result = '공휴일입니다.'
result_detail = '일요일'
else:
result = '공휴일이 아닙니다.'
if month == 1 and day == 1:
result_detail = '신정'
if month == 1 and 21 <= day <= 24:
result_detail = '설 연휴'
if month == 3 and day == 1:
result_detail = '삼일절'
if month == 5 and day == 5:
result_detail = '어린이날'
if month == 5 and day == 27:
result_detail = '석가탄신일'
if month == 6 and day == 6:
result_detail = '현충일'
if month == 8 and day == 15:
result_detail = '광복절'
if month == 9 and 28 <= day <= 30:
result_detail = '추석 연휴'
if month == 10 and day == 3:
result_detail = '개천절'
if month == 10 and day == 9:
result_detail = '한글날'
if month == 12 and day == 25:
result_detail = '성탄절'
if result_detail != '':
print(f'{result_detail} 로 {result}')
else:
print(f'{result}')
귀찮은건 더 도전해보라는 도발에 넘어가 구현해버렸다. 월과 일을 따로 입력받아도 되나 귀찮은걸 싫어하는 유저 편의를 위해 한 번에 입력할 수 있게 구현하였다. 마찬가지로 딕셔너리 + 리스트 구조 형식을 이용했다.
"""
Q2.
1부터 100까지 정수 중 하나를 입력받아
소수(prime number)인지 아닌지 판별해보기
소수면 입력한 값 x는 소수입니다 아니면 소수가 아닙니다 라고 출력하기
"""
if quest_select == 8:
print('\n문제2 (팀명: 똘똘이)')
prime_list = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29,
31, 37, 41, 43, 47, 53, 59, 61, 67,
71, 73, 83, 89, 97]
print('[소수 판별 프로그램]')
user_input_number = int(input('1~100까지의 수 중에서 입력해보세요. '))
if user_input_number in prime_list:
print('소수 입니다.')
else:
print('소수가 아닙니다.')
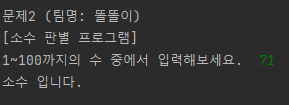
1~100 까지라고? 그럼 바로 'in List' 문법을 사용하기로 했다. 반복 없이 소수 찾기는 너무 고통스럽기 때문에 이렇게 했다.
"""
Q3. 일반승용차 기준
배기량 1000cc 이하 104원/cc
배기량 1600cc 이하 182원/cc
배기량 2000cc 이하 260원/cc
배기량 2500cc 이하 260원/cc
배기량 2500cc 초과 260원/cc
전기 자동차 13만원
차령이 만 3년이 되면 5%가 할인이 되고 매년 5%씩 추가할인이 돼서
만 12년이 되면 최고 50%까지 할인이 됩니다.
1월에 일시납을 하면 6.4%가 추가 할인됩니다.
일반승용차/전기자동차인지 구분하고 일반승용차일 경우
배기량과 차령(차의 연령)을 입력받고 일시납 여부를 묻고 자동차세를 계산해주세요!
원 단위 아래는 절삭합니다.
"""
if quest_select == 9:
print('\n문제3 (팀명: 똘똘이)')
inhale_size = 0
car_age = 0
concede_rate = 1
is_all_paid = False
result_tax = 0
error_flag = False
print('[자동차세 계산 프로그램]')
user_input = int(input('해당 차량의 배기량을 입력하세요\n만약, 전기자동차일 경우엔, 0을 입력하세요.'))
if user_input < 0 or user_input > 10000:
print('잘못된 입력 입니다.')
error_flag = True
elif user_input == 0:
result_tax = 130000
else:
inhale_size = user_input
if inhale_size <= 1000:
result_tax = inhale_size * 104
elif 1000 < inhale_size <= 1600:
result_tax = inhale_size * 182
elif 1600 <= inhale_size <= 2000:
result_tax = inhale_size * 260
elif 2000 <= inhale_size <= 2500:
result_tax = inhale_size * 260
elif 2500 < inhale_size:
result_tax = inhale_size * 260
if error_flag == False:
user_input = int(input('자동차 최초 등록일 기준(자동차등록증 확인) 만으로 몇 년째 입니까'))
if user_input < 0 or user_input > 50:
print('잘못된 입력 입니다.')
error_flag = True
else:
car_age = user_input
if error_flag == False:
user_input = int(input('1월에 자동차세를 한번에 완납하십니까? (추가할인 대상) [1. 예] [2. 아니요] '))
if user_input <= 0 or user_input > 2:
print('잘못된 입력 입니다.')
error_flag = True
else:
is_all_paid = True
if error_flag:
print('계산을 계속 진행할 수 없어 종료합니다.')
else:
if car_age >= 3:
if car_age >= 12:
car_age = 12
concede_rate = (100 - (car_age - 2) * 5) / 100
result_tax = result_tax * concede_rate
if is_all_paid:
result_tax *= concede_rate
result_tax = int(result_tax)
print(f'당신의 올해 자동차세는 {result_tax:10d} 원 입니다.')
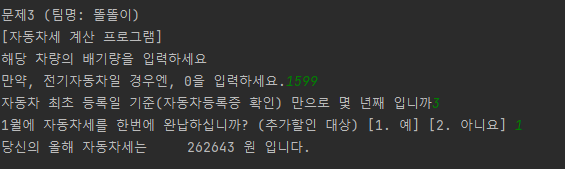
실제로 자동차세를 계산하는 프로그램이었다. 컨셉 재밌어서 구현하면서 몰입하면서 풀었던 기억이 있다.
추가적으로 추가한 기능은 입력 검증 정도만 넣어보았다.
"""
### 문제1 (팀명: 월요일이조 )
위의 데이터를 활용하여 사용자가 주어진 커피를 출력하는 커피머신기를 만들어라.
최대한 딕셔너리 인덱싱과 f스트링을 활용할 것.
조건은 아래와 같다.
# TODO 1. 사용자가 커피 머신을 실행하면 커피머신 상태를 출력한다.
# TODO 2. 사용자에게 '메뉴(아이스아메리카노, 라떼, 플랫화이트)중 하나를 입력하도록 요구한다.
# TODO 3. 커피머신의 재료가 충분한지 비교한다. 충분하면 커피머신의 재료에서 사용자가 주문한 만큼 사용한다. / 충분하지 않으면 '재료가 충분하지 않습니다'라고 출력한다.
# TODO 4. 3번이 충족한다면 사용자에게 금액을 묻는다.
# TODO 5. 금액이 음료가격 이상이라면 잔액을 반환한다. / 금액이 음료가격 미만이라면 '돈이 부족합니다'라고 출력한다.
# TODO 6. 음료를 출력하고 사용자에게 잔액을 반환한다. / 그리고 커피머신 상태를 반환해 준다.
"""
if quest_select == 10:
print('\n문제1 (팀명: 월요일이조)')
drinks = {
"아이스아메리카노": {
"재료": {
'물': 100,
"에스프레소": 50,
'우유': 0,
},
"금액": 1500,
},
"라떼": {
"재료": {
'우유': 150,
"에스프레소": 30,
'물': 0,
},
"금액": 3500,
},
"플랫화이트": {
"재료": {
"우유": 160,
"에스프레소": 40,
'물': 0,
},
"금액": 4000,
},
}
coffee_machine = {
'물': 100,
'에스프레소': 50,
'우유': 150,
}
# TODO 1. 사용자가 커피 머신을 실행하면 커피머신 상태를 출력한다.
print(f"""[우리집 커피머신]
물 : {coffee_machine['물']}ml
에스프레소 : {coffee_machine['에스프레소']}ml
우유 : {coffee_machine['우유']}ml 남음
""")
is_possible_order = True
# TODO 2. 사용자에게 '메뉴(아이스아메리카노, 라떼, 플랫화이트)중 하나를 입력하도록 요구한다.
user_order = int(input('어떤 걸 주문하시겠습니까? [1. 아이스 아메리카노] [2. 라떼] [3. 플랫화이트]'))
if not 1 <= user_order <= 3:
is_possible_order = False
print('가능한 주문이 없습니다. 종료합니다.')
# TODO 3. 커피머신의 재료가 충분한지 비교한다. 충분하면 커피머신의 재료에서 사용자가 주문한 만큼 사용한다. / 충분하지 않으면 '재료가 충분하지 않습니다'라고 출력한다.
if is_possible_order:
if user_order == 1: # 아이스아메리카노
if not ((coffee_machine['물'] >= drinks.get('아이스아메리카노').get('재료').get('물')) and (
coffee_machine['에스프레소'] >= drinks.get('아이스아메리카노').get('재료').get('에스프레소')) and (
coffee_machine['우유'] >= drinks.get('아이스아메리카노').get('재료').get('우유'))):
is_possible_order = False
elif user_order == 2: # 라떼
if not ((coffee_machine['물'] >= drinks.get('라떼').get('재료').get('물')) and (
coffee_machine['에스프레소'] >= drinks.get('라떼').get('재료').get('에스프레소')) and (
coffee_machine['우유'] >= drinks.get('라떼').get('재료').get('우유'))):
is_possible_order = False
elif user_order == 3: # 플랫화이트
if not ((coffee_machine['물'] >= drinks.get('플랫화이트').get('재료').get('물')) and (
coffee_machine['에스프레소'] >= drinks.get('플랫화이트').get('재료').get('에스프레소')) and (
coffee_machine['우유'] >= drinks.get('플랫화이트').get('재료').get('우유'))):
is_possible_order = False
if is_possible_order == False:
print('재료가 충분하지 않아 종료합니다.')
# TODO 4. 3번이 충족한다면 사용자에게 금액을 묻는다.
if is_possible_order:
user_input_money = int(input('얼마를 내시겠습니까?'))
if user_order == 1:
drink_price = drinks.get('아이스아메리카노').get('금액')
if user_input_money < drink_price:
is_possible_order = False
else:
change = user_input_money - drink_price
coffee_machine['물'] -= drinks.get('아이스아메리카노').get('재료').get('물')
coffee_machine['에스프레소'] -= drinks.get('아이스아메리카노').get('재료').get('에스프레소')
coffee_machine['우유'] -= drinks.get('아이스아메리카노').get('재료').get('우유')
if user_order == 2:
drink_price = drinks.get('라떼').get('금액')
if user_input_money < drink_price:
is_possible_order = False
else:
change = user_input_money - drink_price
coffee_machine['물'] -= drinks.get('라떼').get('재료').get('물')
coffee_machine['에스프레소'] -= drinks.get('라떼').get('재료').get('에스프레소')
coffee_machine['우유'] -= drinks.get('라떼').get('재료').get('우유')
if user_order == 3:
drink_price = drinks.get('플랫화이트').get('금액')
if user_input_money < drink_price:
is_possible_order = False
else:
change = user_input_money - drink_price
coffee_machine['물'] -= drinks.get('플랫화이트').get('재료').get('물')
coffee_machine['에스프레소'] -= drinks.get('플랫화이트').get('재료').get('에스프레소')
coffee_machine['우유'] -= drinks.get('플랫화이트').get('재료').get('우유')
# TODO 5. 금액이 음료가격 이상이라면 잔액을 반환한다. / 금액이 음료가격 미만이라면 '돈이 부족합니다'라고 출력한다.
if is_possible_order == True:
print(f'잔돈 {change}원 받으세요')
else:
print('금액이 충분하지 않아 종료합니다.')
# TODO 6. 음료를 출력하고 사용자에게 잔액을 반환한다. / 그리고 커피머신 상태를 반환해 준다.
if is_possible_order:
if user_order == 1:
print('주문하신 아이스아메리카노 나왔습니다.')
elif user_order == 2:
print('주문하신 라떼 나왔습니다.')
elif user_order == 2:
print('주문하신 플랫화이트 나왔습니다.')
print('주문이 완료되었습니다.')
print(f"""[우리집 커피머신]
물 : {coffee_machine['물']}ml
에스프레소 : {coffee_machine['에스프레소']}ml
우유 : {coffee_machine['우유']}ml 남음
""")
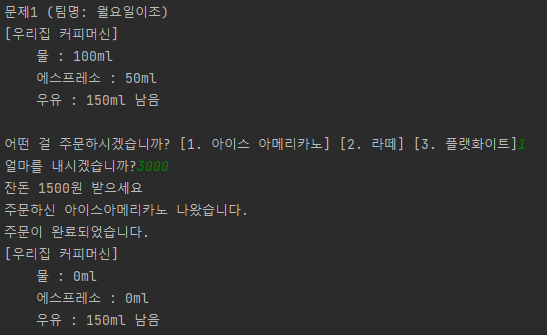
제공된 딕셔너리를 주고 여기서 다시 정해진 양식에 따라 상태를 차감하고 기능을 구현하는 방식이다. 나는 이 문제 아이디어가 마음에 들었던게, 추후에 우리가 공부할 인터페이스와 클래스 개념을 이해할 때 도움이 되기 때문에 좋은 문제라고 생각했다.
옆 부서에서 만든 모듈이 이런 형식으로 데이터를 제공해준다고 하면, 어떻게 사용할 것인가? 이런 제한 조건 안에서 요구된 기능을 수행해야했기 때문에 몰입하면서 풀었다.
"""
### 문제2 (팀명: 월요일이조 )
주어지는 기준 표를 가지고 단위 계산기를 만들어 보세요.
#길이
mm: 1000, cm: 100, m: 1, km: 0.001, in: 39.17, ft: 3.28, yd: 1.09
#넓이
m^2: 1, 평: 0.3025, a: 0.01, ha: 0.0001
#무게
g: 1000, kg: 1, t: 0.001, gr: 15432, oz: 35.27, lb: 2.2
#부피
cc: 1000, dl: 10, l: 1, cm^3: 1000, in^': 61.02, 되: 0.55
#온도
섭씨: 1, 화씨: 33.8, 절대온도: 274.15
각 항목별로 선택을 받아서 기준이 되는 단위와 변환할 단위를 선택해서 출력하게 만들면 됩니다.
"""
if quest_select == 11:
print('\n문제2 (팀명: 월요일이조)')
change_type = ['길이', '넓이', '무게', '부피', '온도']
user_select_num_1 = 0
user_select_num_2 = 0
length_type = {1: ('mm', 1000), 2: ('cm', 100), 3: ('m', 1), 4: ('km', 0.001), 5: ('in', 39.17), 6: ('ft', 3.28),
7: ('yd', 1.09)}
area_type = {1: ('m^2', 1), 2: ('평', 0.3025), 3: ('a', 0.01), 4: ('ha', 0.0001)}
weight_type = {1: ('g', 1000), 2: ('kg', 1), 3: ('t', 0.001), 4: ('gr', 15432), 5: ('oz', 35.27), 6: ('lb', 2.2)}
volume_type = {1: ('cc', 1000), 2: ('dl', 10), 3: ('l', 1), 4: ('cm^3', 1000), 5: ('in^3', 61.02), 6: ('되', 0.55)}
celcius_type = {1: ('섭씨', 1), 2: ('화씨', 33.8), 3: ('절대온도', 274.15)}
message = """변환하고자 하는 타입이 무엇입니까?
[1. 길이]
[2. 넓이]
[3. 무게]
[4. 부피]
[5. 온도]
[타입 선택] : """
user_input_num = int(input(message))
print(f'{change_type[user_input_num - 1]} 를 선택하셨습니다.')
if user_input_num == 1:
message = f"""(1. ) -> (2. ) 어디에서 어디로 바꾸시겠습니까?
1번 항목, 계산하고자하는 값, 그리고 2번 항목을 고르세요.
1. {length_type[1][0]}
2. {length_type[2][0]}
3. {length_type[3][0]}
4. {length_type[4][0]}
5. {length_type[5][0]}
6. {length_type[6][0]}
7. {length_type[7][0]}
"""
elif user_input_num == 2:
message = f"""(1. ) -> (2. ) 어디에서 어디로 바꾸시겠습니까?
1번 항목, 계산하고자하는 값, 그리고 2번 항목을 고르세요.
1. {area_type[1][0]}
2. {area_type[2][0]}
3. {area_type[3][0]}
4. {area_type[4][0]}
"""
elif user_input_num == 3:
message = f"""(1. ) -> (2. ) 어디에서 어디로 바꾸시겠습니까?
1번 항목, 계산하고자하는 값, 그리고 2번 항목을 고르세요.
1. {weight_type[1][0]}
2. {weight_type[2][0]}
3. {weight_type[3][0]}
4. {weight_type[4][0]}
5. {weight_type[5][0]}
6. {weight_type[6][0]}
"""
elif user_input_num == 4:
message = f"""(1. ) -> (2. ) 어디에서 어디로 바꾸시겠습니까?
1번 항목, 계산하고자하는 값, 그리고 2번 항목을 고르세요.
1. {volume_type[1][0]}
2. {volume_type[2][0]}
3. {volume_type[3][0]}
4. {volume_type[4][0]}
5. {volume_type[5][0]}
6. {volume_type[6][0]}
"""
elif user_input_num == 5:
message = f"""(1. ) -> (2. ) 어디에서 어디로 바꾸시겠습니까?
1번 항목, 계산하고자하는 값, 그리고 2번 항목을 고르세요.
1. {celcius_type[1][0]}
2. {celcius_type[2][0]}
3. {celcius_type[3][0]}
"""
print(message)
user_select_num_1 = int(input('[1번 타입 입력] : '))
user_select_num_1_value = int(input('[1번 값 입력] : '))
user_select_num_2 = int(input('[2번 타입 입력] : '))
base = 0
if user_input_num == 1:
base = user_select_num_1_value / length_type[user_select_num_1][1] * length_type[user_select_num_2][1]
message = f'결과값은 {base:f} {length_type[user_select_num_2][0]} 입니다.'
elif user_input_num == 2:
base = user_select_num_1_value / area_type[user_select_num_1][1] * area_type[user_select_num_2][1]
message = f'결과값은 {base:f} {area_type[user_select_num_2][0]} 입니다.'
elif user_input_num == 3:
base = user_select_num_1_value / weight_type[user_select_num_1][1] * weight_type[user_select_num_2][1]
message = f'결과값은 {base:f} {weight_type[user_select_num_2][0]} 입니다.'
elif user_input_num == 4:
base = user_select_num_1_value / volume_type[user_select_num_1][1] * volume_type[user_select_num_2][1]
message = f'결과값은 {base:f} {volume_type[user_select_num_2][0]} 입니다.'
elif user_input_num == 5:
base = user_select_num_1_value / celcius_type[user_select_num_1][1] * celcius_type[user_select_num_2][1]
message = f'결과값은 {base:f} {celcius_type[user_select_num_2][0]} 입니다.'
print(message)
만들면서 개인적으론 너무 고통스러운 문제였다. 방법은 아는데 달리 편하게 할 방법이 없는데, 구현이 복잡한 그런 느낌이 들었다. 또한, 이미 있는걸 만드는 건 또 재미가 없었... 코드를 쓰면서 어떻게 하면 내가 더 편하게 코드를 쓸 수 있을까.. 고민하다보니 "인덱스 안되는 딕셔너리에 인덱스를 넣자"가 만들어졌다.
"""
### 문제3 (팀명: 월요일이조 )
사용자에게서 출생년도를 입력받고 그에 해당하는 결과값을 출력하기
1. 이번달을 기준으로 사용자의 만나이를 구하라
2. 사용자의 띠를 출력하라
3. 사용자에게서 위로 띠동값, 아래로 띠동값인 사람의 각 년생과
현재 나이를 출력하라(이때 나이는 만나이를 고려하지 않는다.)
"""
from datetime import datetime
if quest_select == 12:
print('\n문제3 (팀명: 월요일이조)')
message = """ <만나이, 띠동갑 계산기>
생년월일 6자리를 입력하세요.
"""
birth_year = 0
birth_month = 0
birth_day = 0
today_date = datetime.now()
user_old = 0
user_t = ''
user_t_donggap = []
is_correct_input = True
user_birth_date = input(message)
if len(user_birth_date) != 6:
is_correct_input = False
print('6자리 생년월일이 아니므로 종료합니다.')
if is_correct_input:
if user_birth_date[0] == '0' or user_birth_date[0] == '1' or user_birth_date[0] == '2':
user_birth_year = '20' + user_birth_date[:2]
user_birth_year = int(user_birth_year)
else:
user_birth_year = '19' + user_birth_date[:2]
user_birth_year = int(user_birth_year)
birth_month = int(user_birth_date[2:4])
birth_day = int(user_birth_date[4:6])
# 만나이 계산
user_old = today_date.year - user_birth_year
user_t_index = user_birth_year % 12
# 띠동값 계산
if user_t_index % 12 == 0:
user_t = '원숭이띠'
elif user_t_index % 12 == 1:
user_t = '닭띠'
elif user_t_index % 12 == 2:
user_t = '개띠'
elif user_t_index % 12 == 3:
user_t = '돼지띠'
elif user_t_index % 12 == 4:
user_t = '쥐띠'
elif user_t_index % 12 == 5:
user_t = '소띠'
elif user_t_index % 12 == 6:
user_t = '호랑이띠'
elif user_t_index % 12 == 7:
user_t = '토끼띠'
elif user_t_index % 12 == 8:
user_t = '용띠'
elif user_t_index % 12 == 9:
user_t = '뱀띠'
elif user_t_index % 12 == 10:
user_t = '말띠'
elif user_t_index % 12 == 11:
user_t = '양띠'
# 24살 언더하면서 튜플값 넣어주기
user_t_donggap.append((user_birth_year - 24, user_old + 24))
# 12살 언더하면서 튜플값 넣어주기
user_t_donggap.append((user_birth_year - 12, user_old + 12))
# 12살 어퍼하면서 튜플값 넣어주기
user_t_donggap.append((user_birth_year + 12, user_old - 12))
# 24살 어퍼하면서 튜플값 넣어주기
user_t_donggap.append((user_birth_year + 24, user_old - 24))
print(f'''
현재 일자 기준으로 만으로 {user_old}살이며, {user_t}입니다.
띠동갑은 {user_t_donggap[0][0]}년생 {user_t_donggap[0][1]}살,
띠동갑은 {user_t_donggap[1][0]}년생 {user_t_donggap[1][1]}살,
띠동갑은 {user_t_donggap[2][0]}년생 {user_t_donggap[2][1]}살,
띠동갑은 {user_t_donggap[3][0]}년생 {user_t_donggap[3][1]}살이 있습니다.''')
이번달을 기준으로 해야하는 조건이 있었기 때문에, datetime이라는 내장모듈을 불러와야했다. 어떻게 구현해야할지 금방 생각이 들어서 구현 자체는 어렵지 않았다. 자료 조사를 조금 덜할 수 있게 데이터셋을 같이 제공해줬으면 좋았겠다는 약간의 아쉬움도 있다. 사실 찾는게 어려운건 아니지만, 코딩이 메인이기 때문에 !
"""
### 문제1 (팀명: 경주마팸 )
[ 강의 홍보하기 ]
목표 : 최단시간, 최저비용으로 수강생 20명 만들기
홍보비용 : 100만원
홍보지역 { 북구 : 40명, 광산구 : 35명, 서구 : 30명, 남구 : 20명, 동구 : 10명 }
홍보지역별 비용 { 북구 : 40만원, 광산구 : 35만원, 서구 : 30만원, 남구: 20만원, 동구 : 10만원}
홍보매체 { TV : 30만원, 전단지 : 5만원, 신문 : 15만원, 라디오 : 10만원 , 차량용 스티커 : 20만원 }
홍보기간 : {5일 : 5만원, 10 : 10만원, 15일 : 15만원, 20일 : 20만원, 25일 : 25만원, 30일 : 30만원 }
관심을 가질 확률 { TV : 90%, 전단지 : 60%, 신문 : 70%, 라디오 : 50%, 차량용 스티커 : 80% }
선택한 매체와 지역에서 20명의 수강생이 모집되지 않는다면 실패!
"""
if quest_select == 13:
print('\n문제1 (팀명: 경주마팸) [ 강의 홍보하기 ]')
# TODO : 데이터베이스 정의
location_population = { # 연번 / 지역 / 인구 / 가격
1: ['북구', 40, 40],
2: ['광산구', 35, 35],
3: ['서구', 30, 30],
4: ['남구', 20, 20],
5: ['동구', 10, 10],
}
media_type = { # 연번 / 매체 / 가격 / 관심가질 확률
1: ['TV', 30, 0.9],
2: ['전단지', 5, 0.6],
3: ['신문', 15, 0.7],
4: ['라디오', 10, 0.5],
5: ['차량용 스티커', 20, 0.8]
}
price_of_duration = { # 연번 / 일 기준 / 만원
1: [5, 5],
2: [10, 10],
3: [15, 15],
4: [20, 20],
5: [25, 25],
6: [30, 3]
}
store = {
'location_population': location_population,
'media_type': media_type,
'price_of_duration': price_of_duration,
}
my_budget = 100
# TODO : 상황 설명
message = """<최단 시간 안에 수강생 20명을 만들어라!>
당신은 홍보맨입니다. 홍보 지역, 매체, 기간을 선택하여 수강생 20명을 만들면 됩니다."""
print(message)
# TODO : 홍보 지역 선택
message = f"""
지역 | 거주 인구
1. {store['location_population'][1][0]} \t\t{store['location_population'][1][1]}명
2. {store['location_population'][2][0]} \t\t{store['location_population'][2][1]}명
3. {store['location_population'][3][0]} \t\t{store['location_population'][3][1]}명
4. {store['location_population'][4][0]} \t\t{store['location_population'][4][1]}명
5. {store['location_population'][5][0]} \t\t{store['location_population'][5][1]}명
홍보 지역을 선택하세요."""
print(message)
user_select_location = int(input('[번호 입력] : '))
# TODO : 홍보 매체 선택
message = f"""
홍보 매체\t|\t비용
1. {store['media_type'][1][0]} \t\t\t\t{store['media_type'][1][1]} 만원
2. {store['media_type'][2][0]} \t\t\t{store['media_type'][2][1]} 만원
3. {store['media_type'][3][0]} \t\t\t{store['media_type'][3][1]} 만원
4. {store['media_type'][4][0]} \t\t\t{store['media_type'][4][1]} 만원
5. {store['media_type'][5][0]} \t{store['media_type'][5][1]} 만원
홍보 지역을 선택하세요."""
print(message)
user_select_media = int(input('[번호 입력] : '))
# TODO : 홍보 기간 선택
message = f"""
홍보 기간\t|\t비용
1. {store['price_of_duration'][1][0]}일 당 \t\t\t{store['price_of_duration'][1][1]} 만원
2. {store['price_of_duration'][2][0]}일 당 \t\t\t{store['price_of_duration'][2][1]} 만원
3. {store['price_of_duration'][3][0]}일 당 \t\t\t{store['price_of_duration'][3][1]} 만원
4. {store['price_of_duration'][4][0]}일 당 \t\t\t{store['price_of_duration'][4][1]} 만원
5. {store['price_of_duration'][5][0]}일 당 \t\t\t{store['price_of_duration'][5][1]} 만원
홍보 기간을 선택하세요."""
print(message)
user_select_duration = int(input('[번호 입력] : '))
# TODO : 홍보 매체에 따른 예상 관심 인원 산출
# 인구 = 지역별 인구 * 매체별 어트랙션 -> 정수화
population = store.get('location_population')[user_select_location][1] \
* store.get('media_type')[user_select_media][2]
population = int(population)
# 비용 = 지역별 비용 + 매체별 어트랙션 + 기간
total_cost = store.get('location_population')[user_select_location][2] \
+ store.get('media_type')[user_select_media][1] \
+ store.get('price_of_duration')[user_select_duration][1]
# TODO : 성공 조건 여부 검증
is_cost_verified = False
is_contract_verified = False
if population >= 20:
is_contract_verified = True
else:
is_contract_verified = False
if total_cost <= my_budget:
is_cost_verified = True
else:
is_cost_verified = False
# TODO : 결과 화면 출력
append_message = ''
if is_contract_verified:
append_message = append_message + f'수강생 20명 이상 달성하기 [성공!]\n 수강 인원 : {population} 명\n'
else:
append_message = append_message + f'수강생 20명 이상 달성하기 [실패!]\n 수강 인원 : {population} 명\n'
if is_cost_verified:
append_message = append_message + f'예산 {my_budget}만원 이하 달성하기 [성공!]\n 소요 예산 : {total_cost} 만원\n'
else:
append_message = append_message + f'예산 {my_budget}만원 이하 달성하기 [실패!]\n 소요 예산 : {total_cost} 만원\n'
print(append_message)
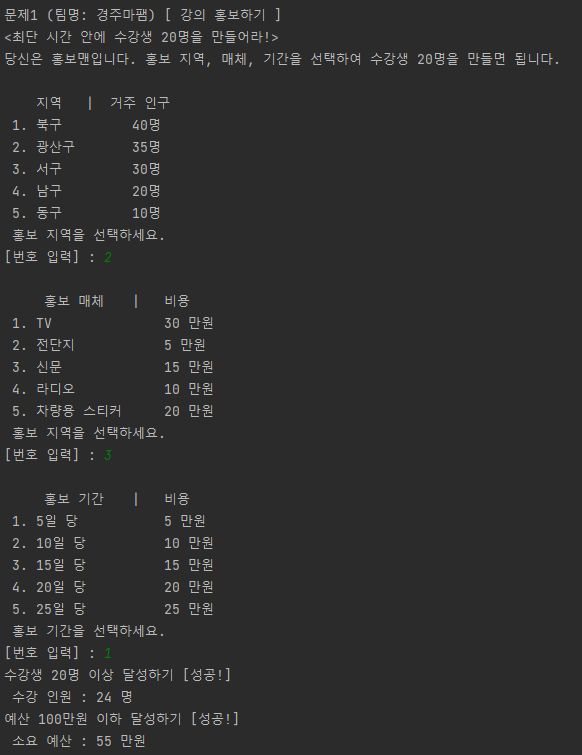
처음에 제공된 데이터셋을 내가 압축시켜 'store'라는 딕셔너리 자료형으로 만들었다. 처음에 문제를 읽고 무슨 뜻인지 몰라서 다른 사람의 풀이를 어느정도 보고 풀이 방법을 알게 되었다. 처음에 price는 홍보 매체 와 기간을 통해 정해지는게 아닌가? 홍보 기간에 따라 랜덤으로 수강생들이 신청을 하게되는 시뮬레이션 문제인가? 그래서 가격은 왜 매체별로 다르지 않고 같은가? 점점 오리무중에 들어갔다가 단순한 계산 문제라는걸 알고 금방 풀 수 있게 되었다. 제공된 자료형을 손질하고 정리하는 방법을 터득한 좋은 문제라고 생각한다.
"""
### 문제2 (팀명: 경주마팸 )
[ 아이템 장착하기 ]
공격력, 방어력, 스피드는 각자 5의 값을 가집니다.
기존 아이템 정보를 사용하셔도됩니다, item 이름 추가 및 스텟 추가 가능합니다,
인벤토리및 착용상태를 출력할때 아이템 이름이 나오도록 구현하세요.
inventory안의 item을 사용할때 inventory에서 item이사라지게하고
착용상태에서 item이 나오고 item의 옵션이 적용되도록 구현
스텟의 증가옵션은 자율적으로 구현
마지막 결과창에 인벤토리, 착용상태, 스텟 상태 출력
"""
if quest_select == 14:
print('\n문제2 (팀명: 경주마팸) [ 아이템 장착하기 ]')
# 인벤토리 자료형
wood_helmet = {
'name': 'wood helmet',
'type': 'head_armor',
'effect_armor': 2,
}
steel_helmet = {
'name': 'steel helmet',
'type': 'head_armor',
'effect_armor': 3,
'effect_speed': -1
}
wood_sword = {
'name': 'wood sword',
'type': 'melee_weapon',
'effect_attack': 2,
'effect_speed': 3,
}
long_sword = {
'name': 'long sword',
'type': 'melee_weapon',
'effect_attack': 5,
'effect_speed': -3,
}
wood_shield = {
'name': 'wood shield',
'type': 'barrier',
'effect_armor': 3,
}
steel_shield = {
'name': 'steel shield',
'type': 'barrier',
'effect_armor': 5,
'effect_speed': -1,
}
doping_portion_attack = {
'name': 'doping portion attack',
'type': 'spend_item',
'effect_attack': 7,
}
void_item = {
'name': '없음',
'type': '',
}
inventory = {
'slot1': wood_helmet,
'slot2': steel_helmet,
'slot3': wood_sword,
'slot4': long_sword,
'slot5': wood_shield,
'slot6': steel_shield,
'slot7': doping_portion_attack
}
status = {
'attack': 5,
'armor': 5,
'speed': 5
}
equipment = {
"head_armor": void_item,
"melee_weapon": void_item,
"barrier": void_item,
}
user = {
'status': status,
'inventory': inventory,
'equipment': equipment
}
# 유저 스탯 확인
status_message = f"""< 유저 스테이터스 >
* 공격력 {user.get('status').get('attack')}
* 방어력 {user.get('status').get('armor')}
* 스피드 {user.get('status').get('speed')}
< 착용 중 물건 >
* 머리 {user.get('equipment').get('head_armor').get('name')}
* 손 {user.get('equipment').get('melee_weapon').get('name')}
* 방패 {user.get('equipment').get('barrier').get('name')}"""
print(status_message)
print("""무슨 행동을 할까요?
1. 인벤토리 확인""")
user_input_num = int(input('[번호 입력] : '))
if user_input_num == 1:
# 인벤토리 보여주기
inventory_message = f"""[나의 인벤토리]
* 1번 슬롯 : {user.get('inventory').get('slot1').get('name')}
* 2번 슬롯 : {user.get('inventory').get('slot2').get('name')}
* 3번 슬롯 : {user.get('inventory').get('slot3').get('name')}
* 4번 슬롯 : {user.get('inventory').get('slot4').get('name')}
* 5번 슬롯 : {user.get('inventory').get('slot5').get('name')}
* 6번 슬롯 : {user.get('inventory').get('slot6').get('name')}
* 7번 슬롯 : {user.get('inventory').get('slot7').get('name')}"""
print(inventory_message)
# 착용할지 여부 묻기
user_want_use = int(input('착용 또는 사용을 원합니까? [1. 네] [2. 아니요] '))
# 착용한다고 하면 추가하기
if user_want_use == 1:
user_using_slot_num = int(input('몇 번 슬롯의 아이템을 사용 또는 착용할까요? '))
# 타입에 따라 착용 부위 달라짐, 인벤토리 썼으면 소모시키기
if user_using_slot_num == 1:
selected_item = user.get('inventory').get('slot1')
user.get('inventory')['slot1'] = void_item
if user_using_slot_num == 2:
selected_item = user.get('inventory').get('slot2')
user.get('inventory')['slot2'] = void_item
if user_using_slot_num == 3:
selected_item = user.get('inventory').get('slot3')
user.get('inventory')['slot3'] = void_item
if user_using_slot_num == 4:
selected_item = user.get('inventory').get('slot4')
user.get('inventory')['slot4'] = void_item
if user_using_slot_num == 5:
selected_item = user.get('inventory').get('slot5')
user.get('inventory')['slot5'] = void_item
if user_using_slot_num == 6:
selected_item = user.get('inventory').get('slot6')
user.get('inventory')['slot6'] = void_item
if user_using_slot_num == 7:
selected_item = user.get('inventory').get('slot7')
user.get('inventory')['slot7'] = void_item
user.get('equipment')[selected_item.get('type')] = selected_item
if selected_item.get('effect_attack') is not None:
user.get('status')['attack'] += selected_item.get('effect_attack')
if selected_item.get('effect_armor') is not None:
user.get('status')['armor'] += selected_item.get('effect_armor')
if selected_item.get('effect_speed') is not None:
user.get('status')['speed'] += selected_item.get('effect_speed')
# 착용 안한다고 하면 패스
elif user_want_use == 2:
pass
# 변경된 스탯 보여주기
status_message = f"""< 유저 스테이터스 >
* 공격력 {user.get('status').get('attack')}
* 방어력 {user.get('status').get('armor')}
* 스피드 {user.get('status').get('speed')}
< 착용 중 물건 >
* 머리 {user.get('equipment').get('head_armor').get('name')}
* 손 {user.get('equipment').get('melee_weapon').get('name')}
* 방패 {user.get('equipment').get('barrier').get('name')}"""
print(status_message)
inventory_message = f"""[나의 인벤토리]
* 1번 슬롯 : {user.get('inventory').get('slot1').get('name')}
* 2번 슬롯 : {user.get('inventory').get('slot2').get('name')}
* 3번 슬롯 : {user.get('inventory').get('slot3').get('name')}
* 4번 슬롯 : {user.get('inventory').get('slot4').get('name')}
* 5번 슬롯 : {user.get('inventory').get('slot5').get('name')}
* 6번 슬롯 : {user.get('inventory').get('slot6').get('name')}
* 7번 슬롯 : {user.get('inventory').get('slot7').get('name')}"""
print(inventory_message)
print('종료합니다.')
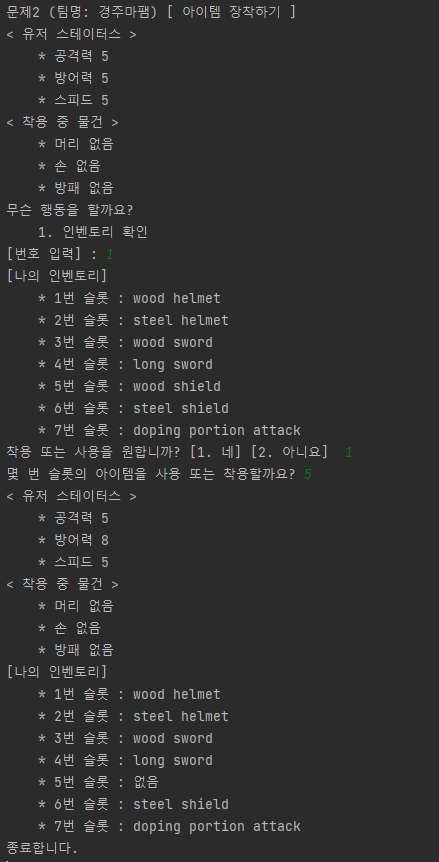
추후에 배우게될 객체지향적인 느낌으로 소스를 구상해보았다. 단순히 ['wood helmet', 0, 2, 0 ] 이렇게 구성해도 됐지만, 게임이기도 하고, 인벤토리에서 넣고, 빼고 하는 기능의 느낌을 구현하기 위해 일일히 객체를 하나씩 만들어주어야했다. 추가적으로 포션도 만들어보았다. '없음'이라는 단어를 만들기 위해 void_item도 만들어 같은 텍스트 출력 소스더라도 기능이 가능하게 만들었다. 이 코드를 쓰면서... 클래스.. 상속.. 함수.. 마려웠다. 좋은 문제라고 생각합니다.
"""
### 문제3 (팀명: 경주마팸 )
[ 내생에 마지막 다이어트 ]
1. 성별, 나이, 몸무게 입력 → BMI 알려주기 ( 목표 체중 )
2. 운동 10가지 중 한가지 필수 선택
3. 운동후 근손실 방지를 위해 식품섭취는 필수 최소 1개 이상.
4. 운동 소모 칼로리, 음식 섭취 칼로리 계산해서 다이어트 몇일 걸리는지 알려주기
살이 빠지려면 운동 9000kcal소모시 1kg감량됨.
추가 음식 섭취시 9000kcal섭취하면 1kg증량됨
"""
if quest_select == 15:
print('\n문제3 (팀명: 경주마팸) [ 내생에 마지막 다이어트 ]')
# TODO: 데이터 자료화
work_out_data = {
1: ['걷기', 188],
2: ['자전거', 395],
3: ['요가', 123],
4: ['줄넘기', 494],
5: ['수영', 444],
6: ['계단', 345],
7: ['등산', 395],
8: ['복싱', 494],
9: ['달리기', 345],
10: ['에어로빅', 296],
}
food_data = {
1: ['김치찌게', 7.57, 6.47, 7.51, 124.04],
2: ['떡볶이', 2.91, 60.42, 6.97, 295.75],
3: ['치킨(1조각)', 14.85, 8.58, 19.19, 244.73],
4: ['피자(1조각)', 10.48, 26.08, 10.7, 241.44],
5: ['삼겹살', 56.43, 1.18, 34.47, 650.47],
6: ['야채죽', 0.59, 28.8, 6.1, 144.91],
7: ['치킨샐러드', 31.5, 2.55, 29.48, 411.62],
8: ['햄버거', 9.77, 34.25, 12.32, 274.21],
}
# TODO: 키, 몸무게 입력 받기
user_height = int(input('키를 입력하세요.(cm기준) '))
user_weight = int(input('몸무게를 입력하세요.(kg) '))
# TODO: BMI, 목표 체중 보여주기
standard_weight = ((user_height / 100) ** 2) * 23
now_bmi = user_weight / ((user_height / 100) ** 2)
target_decreasing_weight = user_weight - standard_weight
is_possible_state = True
if target_decreasing_weight < 0:
print('다이어트 할 필요가 없습니다. 종료합니다.')
is_possible_state = False
if is_possible_state:
print(f"""현재 bmi 는 {now_bmi:.1f} 입니다.
감량해야하는 무게는 {target_decreasing_weight:.1f}kg 입니다.
어떤 운동을 계획하고 있습니까?
운동 타입 소모 칼로리
1. {work_out_data[1][0]} \t\t\t{work_out_data[1][1]} kcal/hr
2. {work_out_data[2][0]} \t\t{work_out_data[2][1]} kcal/hr
3. {work_out_data[3][0]} \t\t\t{work_out_data[3][1]} kcal/hr
4. {work_out_data[4][0]} \t\t{work_out_data[4][1]} kcal/hr
5. {work_out_data[5][0]} \t\t\t{work_out_data[5][1]} kcal/hr
6. {work_out_data[6][0]} \t\t\t{work_out_data[6][1]} kcal/hr
7. {work_out_data[7][0]} \t\t\t{work_out_data[7][1]} kcal/hr
8. {work_out_data[8][0]} \t\t\t{work_out_data[8][1]} kcal/hr
9. {work_out_data[9][0]} \t\t{work_out_data[9][1]} kcal/hr
10. {work_out_data[10][0]} \t\t{work_out_data[10][1]} kcal/hr
""")
# TODO: 목표 체중까지 운동할지 선택
user_select_work_out_type = int(input('어떤 운동을 할 예정입니까?'))
# TODO: 하루 평균 몇 시간?
user_select_work_out_hour = int(input('하루 평균 몇 시간을 할 예정입니까?'))
# TODO: 목표 일수 계산
predict_work_out_days = 9000 * target_decreasing_weight / (
work_out_data[user_select_work_out_type][1] * user_select_work_out_hour)
predict_work_out_days = int(predict_work_out_days)
print(f"""예상 목표 기간은 {predict_work_out_days}일 입니다.""")
# TODO: 치팅 데이가 있습니까?
is_cheating_exist = False
user_answer = int(input('치팅 데이가 있습니까? [1. 네] [2. 아니요] '))
if user_answer == 1:
is_cheating_exist = True
# TODO: 치팅 데이 간격을 얼마나?
cheat_term = int(input('치팅 데이 간격을 얼마나 둘 예정입니까? (예시 : 1주일마다 1번이면 7 입력) '))
# TODO: 치팅 데이 메뉴?
cheating_food_list_message = f"""어떤 음식으로 드실 예정입니까?
1. {food_data[1][0]} {int(food_data[1][4])}kcal (1인분)
2. {food_data[2][0]} {int(food_data[2][4])}kcal (1인분)
3. {food_data[3][0]} {int(food_data[3][4])}kcal (1인분)
4. {food_data[4][0]} {int(food_data[4][4])}kcal (1인분)
5. {food_data[5][0]} {int(food_data[5][4])}kcal (1인분)
6. {food_data[6][0]} {int(food_data[6][4])}kcal (1인분)
7. {food_data[7][0]} {int(food_data[7][4])}kcal (1인분)"""
print(cheating_food_list_message)
cheat_type = int(input('[번호 입력]'))
# TODO: 치팅 데이 식사량 얼마나?
cheat_portion = int(input('몇 인분 먹습니까? (예시 : 1인분이면 1 입력) '))
increase_kcal = (food_data[cheat_type][4] * cheat_portion) * (predict_work_out_days / cheat_term)
increase_days = increase_kcal / (work_out_data[user_select_work_out_type][1] * user_select_work_out_hour)
increase_days = int(increase_days)
# TODO: 치팅 데이 포함시 예상기간은 얼마나?
print(
f"""예상 목표 기간은 {predict_work_out_days}일에서 {increase_days} 일 늘어나 {predict_work_out_days + increase_days}일이 걸릴 예정 입니다.""")
print('다이어트에 꼭 성공하십시요. 무운을 빕니다.')
살다보면 제공받은 데이터셋이 늘 완성되지 않은 상태일 수 있다! 그러면 누가 원하는 데이터셋을 만들어줄 때까지 요청하고 기다리는 것보단, 자기가 할 수 있으면 만들어버리는 것도 방법이다. 제공된 데이터셋의 영양분석표를 통해 칼로리를 계산하였고, 치팅데이 간격만큼 해당 칼로리를 더하여 '며칠이 더 늘어나는지' 추가 정보를 제공하였다. 다만, 기본 제공된 데이터에서 지방 1kg은 9000kcal 인데, 1000kcal이라고 올려주셨다. 나도 늦게 발견해서 그냥 가만히 있었다. 깔끔하게 코딩하신 분들은 금방 수정하실 수 있을 것이고, 안그런 사람들은 어떻게 하면 자기가 쓴 코드를 손 많이 안대고 수정할 수 있을지.. ? 고민해보고 왜 깔끔한 코딩을 추구하려고 해야하는지 체감하게 될 일이니깐..
"""
### 문제1 (팀명: 김주김 )
1번. 놀이기구 추천
당신은 놀이기구를 추천해주는 로봇을 만들어야 합니다. 입장객의 상태를 입력받아 주어진 조건을 만족하는 코드를 짜세요.
1) 롤러코스터는 속도가 가장 빠른 놀이기구 입니다. 키 130cm이하는 탑승할 수 없습니다.
2) 공중그네는 높고 빠르지만 키와 나이 제한이 없습니다.
3) 자이로드롭은 가장 높이 올라가는 놀이기구 입니다. 키 130cm이하 및 만6세 미만은 탑승할 수 없습니다.
4) 귀신의 집은 어린 아이들 정서에 좋지 않습니다.
5) 회전목마는 누구나 즐길 수 있습니다.
"""
if quest_select == 16:
print('\n문제1 (팀명: 김주김) 놀이기구 추천')
# 입력 받을 자료 : 입장객 나이, 키
# 추천 범위 : 롤러코스터, 공중그네, 자이로드롭, 귀신의집, 회전목마
message = """<놀이기구 추천 봇>
안녕하세요. 놀이기구 추천 봇입니다.
입장객의 키와 나이를 입력해주세요."""
print(message)
height = int(input('[키 입력] : '))
age = int(input('[만 나이 입력] : '))
append_message = ''
# 롤러코스터 확인
if height > 130:
append_message = append_message + """ [놀이기구 국룰 원탑를 원한다면?]
롤러코스터\n"""
# 공중그네 확인
append_message = append_message + """ [빠른 속도감과 안정적인 놀이기구 맛를 원한다면?]
공중그네\n"""
# 자이로드롭 확인
if height > 130 and age > 6:
append_message = append_message + """ [다이나믹한 놀이기구를 원한다면?]
자이로드롭\n"""
# 귀신의집 확인
if age > 12:
append_message = append_message + """ [오싹오싹한 체험을 원한다면?]
귀신의집\n"""
# 회전목마 확인
append_message = append_message + """ [편안한 즐거움을 원한다면?]
회전목마"""
result_message = f"""
입력하신 정보를 바탕으로 다음과 같은 놀이기구 탑승을 제안합니다.\n{append_message}"""
# 결과 출력
print(result_message)
조건을 이용해 놀이기구를 제안해주는 문제였다. 데이터만 잘 활용하면 결론이나 추천을 내려주는 '전문가 시스템'의 모티브가 될 수 있는 좋은 문제라고 생각했다.
"""
### 문제2 (팀명: 김주김 )
2번. 간식 구매
1) 입장권의 종류는 입장권(기구 탑승 불가), 일반 탑승권, 슈퍼패스권이 있습니다.
2) 입장권의 종류에 따라 구매 가능한 간식의 수량이 다릅니다.
3) 간식의 종류는 츄러스, 솜사탕, 와플, 구슬아이스크림가 있습니다.
4) 슈퍼패스권 입장객은 간식 구매시 무료 에이드 한잔 혹은 총 구매액의 5%할인 중 선택 할 수 있습니다.
5) 일반 탑승권 입장객은 한 번 구매시 최대 3개까지 가능합니다.
6) 입장권만 가진 입장객은 한번에 한 메뉴만 구매 가능합니다.
7) 각 간식의 가격은 임의로 설정하며 구매한 내역과 결제금액을 마지막에 출력합니다.
"""
if quest_select == 17:
print('\n문제2 (팀명: 김주김) 간식 구매')
entrance_structure = {
'입장권': [(1, '기본 입장권'), (2, '일반 탑승권'), (3, '슈퍼패스권')],
'간식 종류': [(1, '츄러스', 2000), (2, '솜사탕', 1500), (3, '와플', 3000), (4, '구슬아이스크림', 4000)]
}
# TODO : 변수 선언
user_entrance_type = 0 # 입장권 저장
user_buy_list = [] # 구매 리스트 저장
is_possible_order = True
is_needed_discount = False
buyable_count = 0
message = \
f"""
본인의 입장권을 선택하세요
[{entrance_structure.get('입장권')[0][0]}. {entrance_structure.get('입장권')[0][1]}]
[{entrance_structure.get('입장권')[1][0]}. {entrance_structure.get('입장권')[1][1]}]
[{entrance_structure.get('입장권')[2][0]}. {entrance_structure.get('입장권')[2][1]}]
[번호 입력] : """
# TODO : 유저 입장권 확인
user_input = int(input(message))
# 입력 확인
if user_input < 1 or user_input > 3:
is_possible_order = False
print('비정상적인 입력입니다. 다시 실행해주세요.')
if is_possible_order:
user_entrance_type = user_input
message = f"""[{entrance_structure.get('입장권')[user_entrance_type - 1][1]}]를 선택하셨습니다."""
print(message)
# TODO : 일반 탑승시 3개까지 구매 가능
# TODO : 기본 입장권은 1개만 구매 가능
if user_entrance_type == 1:
buyable_count = 1
else:
buyable_count = 3
# TODO : 슈퍼패스 시 무료 1잔 선택할 시, 한잔 주고 바로 종료. 아니면, 구매액 5% 할인 3개까지
if user_entrance_type == 3:
message = """ 슈퍼패스는 다음 둘 중의 하나를 선택할 수 있습니다.
[1. 무료 에이드 1잔]
[2. 간식 구매 시 5% 할인]
[번호 입력] : """
user_input = int(input(message))
if user_input == 1:
print('여기 에이드 있습니다. [주문 종료!]')
is_possible_order = False
elif user_input == 2:
is_needed_discount = True
else:
is_possible_order = False
print('비정상적인 입력입니다. 다시 실행해주세요.')
if is_possible_order:
# TODO : 간식 종류 : 츄러스, 솜사탕, 와플, 구슬아이스크림
if buyable_count > 0:
message = \
f"""
간식의 종류는 다음과 같습니다. 선택 가능 : {buyable_count} 개 남음
[{entrance_structure.get('간식 종류')[0][0]}. {entrance_structure.get('간식 종류')[0][1]} {entrance_structure.get('간식 종류')[0][2]}원]
[{entrance_structure.get('간식 종류')[1][0]}. {entrance_structure.get('간식 종류')[1][1]} {entrance_structure.get('간식 종류')[1][2]}원]
[{entrance_structure.get('간식 종류')[2][0]}. {entrance_structure.get('간식 종류')[2][1]} {entrance_structure.get('간식 종류')[2][2]}원]
[{entrance_structure.get('간식 종류')[3][0]}. {entrance_structure.get('간식 종류')[3][1]} {entrance_structure.get('간식 종류')[3][2]}원]
[번호 입력] : """
user_input = int(input(message))
if user_input < 1 or user_input > 4:
is_possible_order = False
print('비정상적인 입력입니다. 다시 실행해주세요.')
else:
user_buy_list.append(entrance_structure.get('간식 종류')[user_input - 1])
buyable_count -= 1
if buyable_count > 0 and is_possible_order:
message = \
f"""
간식의 종류는 다음과 같습니다. 선택 가능 : {buyable_count} 개 남음
[{entrance_structure.get('간식 종류')[0][0]}. {entrance_structure.get('간식 종류')[0][1]} {entrance_structure.get('간식 종류')[0][2]}원]
[{entrance_structure.get('간식 종류')[1][0]}. {entrance_structure.get('간식 종류')[1][1]} {entrance_structure.get('간식 종류')[1][2]}원]
[{entrance_structure.get('간식 종류')[2][0]}. {entrance_structure.get('간식 종류')[2][1]} {entrance_structure.get('간식 종류')[2][2]}원]
[{entrance_structure.get('간식 종류')[3][0]}. {entrance_structure.get('간식 종류')[3][1]} {entrance_structure.get('간식 종류')[3][2]}원]
[번호 입력] : """
user_input = int(input(message))
if user_input < 1 or user_input > 4:
is_possible_order = False
print('비정상적인 입력입니다. 다시 실행해주세요.')
else:
user_buy_list.append(entrance_structure.get('간식 종류')[user_input - 1])
buyable_count -= 1
if buyable_count > 0 and is_possible_order:
message = \
f"""
간식의 종류는 다음과 같습니다. 선택 가능 : {buyable_count} 개 남음
[{entrance_structure.get('간식 종류')[0][0]}. {entrance_structure.get('간식 종류')[0][1]} {entrance_structure.get('간식 종류')[0][2]}원]
[{entrance_structure.get('간식 종류')[1][0]}. {entrance_structure.get('간식 종류')[1][1]} {entrance_structure.get('간식 종류')[1][2]}원]
[{entrance_structure.get('간식 종류')[2][0]}. {entrance_structure.get('간식 종류')[2][1]} {entrance_structure.get('간식 종류')[2][2]}원]
[{entrance_structure.get('간식 종류')[3][0]}. {entrance_structure.get('간식 종류')[3][1]} {entrance_structure.get('간식 종류')[3][2]}원]
[번호 입력] : """
user_input = int(input(message))
if user_input < 1 or user_input > 4:
is_possible_order = False
print('비정상적인 입력입니다. 다시 실행해주세요.')
else:
user_buy_list.append(entrance_structure.get('간식 종류')[user_input - 1])
buyable_count -= 1
if is_possible_order:
# TODO : 구매 내역, 결제 금액 정리
order_result = {
entrance_structure['간식 종류'][0][1]: 0,
entrance_structure['간식 종류'][1][1]: 0,
entrance_structure['간식 종류'][2][1]: 0,
entrance_structure['간식 종류'][3][1]: 0,
"total_price": 0
}
# TODO : 구매 내역, 결제 금액 출력
if len(user_buy_list) > 0:
temp_pop = user_buy_list.pop()
# temp_pop 예시 : (1, '츄러스', 2000)
order_result[temp_pop[1]] += 1
order_result["total_price"] += temp_pop[2]
if len(user_buy_list) > 0:
temp_pop = user_buy_list.pop()
order_result[temp_pop[1]] += 1
order_result["total_price"] += temp_pop[2]
if len(user_buy_list) > 0:
temp_pop = user_buy_list.pop()
order_result[temp_pop[1]] += 1
order_result["total_price"] += temp_pop[2]
if user_entrance_type == 3:
order_result["total_price"] *= 0.95
order_result["total_price"] = int(order_result["total_price"])
append_message = ''
if order_result[entrance_structure['간식 종류'][0][1]] > 0:
append_message = append_message + f"\t{entrance_structure['간식 종류'][0][1]} {order_result[entrance_structure['간식 종류'][0][1]]} 개\n"
if order_result[entrance_structure['간식 종류'][1][1]] > 0:
append_message = append_message + f"\t{entrance_structure['간식 종류'][1][1]} {order_result[entrance_structure['간식 종류'][1][1]]} 개\n"
if order_result[entrance_structure['간식 종류'][2][1]] > 0:
append_message = append_message + f"\t{entrance_structure['간식 종류'][2][1]} {order_result[entrance_structure['간식 종류'][2][1]]} 개\n"
if order_result[entrance_structure['간식 종류'][3][1]] > 0:
append_message = append_message + f"\t{entrance_structure['간식 종류'][3][1]} {order_result[entrance_structure['간식 종류'][3][1]]} 개\n"
message = f"""
[구매 물품 목록]
주문목록 :
{append_message}
총 가격 : {order_result["total_price"]} 원
[주문 종료!]
"""
print(message)
복잡한 조건을 제공하고, 이를 실제로 구현하도록 하는 문제였다. 텍스트가 많기는 했지만, 그래도 객체지향적으로 프로그램이 구동되도록 노력해보았다. 그러면? entrance_structure 내부의 데이터만 수정해주어도 이름과 가격을 수정해줄 수 있다. 다만, 아직 반복문과 함수가 없어 완전한 상태는 아니라는걸 참고해주면 좋겠다.
"""
### 문제3 (팀명: 김주김 )
3번. 편의점 뒷풀이
1) 편의점에서 판매하고 있는 상품은 다음과 같습니다.
마른오징어, 초코우유, 삼각김밥, 감자칩, 버터쿠키, 감동란
처음처럼, 진로, 카스, 기네스, 서머스비
2) 무조건 5개의 상품을 구매합니다.
3) 구매 목록에 주류가 있는 경우, 성인 확인을 진행합니다.
4) 미성년자인 경우 주류를 제외한 상품만 구매 가능합니다.
5) 결제 결과를 출력하세요.
"""
if quest_select == 18:
print('\n문제3 (팀명: 김주김) 편의점 뒷풀이')
database = { # '상품명', '가격', '성인구매가능 상품'
'상품': {1: ['마른오징어', 1200, False],
2: ['초코우유', 1000, False],
3: ['삼각김밥', 900, False],
4: ['감자칩', 1500, False],
5: ['버터쿠키', 1600, False],
6: ['감동란', 1600, False],
7: ['처음처럼', 1600, True],
8: ['진로', 1600, True],
9: ['카스', 2500, True],
10: ['기네스', 2500, True],
11: ['서머스비', 2500, True]}
}
user_select_list = []
order_result = {
1: 0,
2: 0,
3: 0,
4: 0,
5: 0,
6: 0,
7: 0,
8: 0,
9: 0,
10: 0,
11: 0,
'total_price': 0
}
# 1회차
message = f"""
편의점 상품 리스트
1. {database['상품'][1][0]} {database['상품'][1][1]}원
2. {database['상품'][2][0]} {database['상품'][2][1]}원
3. {database['상품'][3][0]} {database['상품'][3][1]}원
4. {database['상품'][4][0]} {database['상품'][4][1]}원
5. {database['상품'][5][0]} {database['상품'][5][1]}원
6. {database['상품'][6][0]} {database['상품'][6][1]}원
7. {database['상품'][7][0]} {database['상품'][7][1]}원
8. {database['상품'][8][0]} {database['상품'][8][1]}원
9. {database['상품'][9][0]} {database['상품'][9][1]}원
10. {database['상품'][10][0]} {database['상품'][10][1]}원
11. {database['상품'][11][0]} {database['상품'][11][1]}원"""
print(message)
select_num = int(input('[구매할 상품 번호 입력] : '))
is_possible_order = True
if select_num < 1 or select_num >= len(database['상품']):
is_possible_order = False
print('잘못된 번호를 입력했습니다. 프로그램 종료함!')
if is_possible_order:
# 옳은 번호 입력, 리스트에 상품 추가
user_select_list.append(database['상품'][select_num])
order_result[select_num] += 1
# 2회차
if is_possible_order:
print(message)
select_num = int(input('[구매할 상품 번호 입력] : '))
if select_num < 1 or select_num >= len(database['상품']):
is_possible_order = False
print('잘못된 번호를 입력했습니다. 프로그램 종료함!')
if is_possible_order:
user_select_list.append(database['상품'][select_num])
order_result[select_num] += 1
# 3회차
if is_possible_order:
print(message)
select_num = int(input('[구매할 상품 번호 입력] : '))
if select_num < 1 or select_num >= len(database['상품']):
is_possible_order = False
print('잘못된 번호를 입력했습니다. 프로그램 종료함!')
if is_possible_order:
user_select_list.append(database['상품'][select_num])
order_result[select_num] += 1
# 4회차
if is_possible_order:
print(message)
select_num = int(input('[구매할 상품 번호 입력] : '))
if select_num < 1 or select_num >= len(database['상품']):
is_possible_order = False
print('잘못된 번호를 입력했습니다. 프로그램 종료함!')
if is_possible_order:
user_select_list.append(database['상품'][select_num])
order_result[select_num] += 1
# 5회차
if is_possible_order:
print(message)
select_num = int(input('[구매할 상품 번호 입력] : '))
if select_num < 1 or select_num >= len(database['상품']):
is_possible_order = False
print('잘못된 번호를 입력했습니다. 프로그램 종료함!')
if is_possible_order:
user_select_list.append(database['상품'][select_num])
order_result[select_num] += 1
# 5회차 종료, 최종 리스트에서 True가 있는가? 있으면 성인인증
order_result['total_price'] += user_select_list[0][1]
order_result['total_price'] += user_select_list[1][1]
order_result['total_price'] += user_select_list[2][1]
order_result['total_price'] += user_select_list[3][1]
order_result['total_price'] += user_select_list[4][1]
if True in [user_select_list[0][2], user_select_list[1][2], user_select_list[2][2], user_select_list[3][2],
user_select_list[4][2]]:
print('성인 인증을 시행합니다.')
age = int(input('몇 살 입니까? '))
if age < 19:
print('구매 불가한 상품이 있습니다. 종료합니다.')
is_possible_order = False
append_message = ''
if order_result[1] > 0:
append_message = append_message + f"\t*{database['상품'][1][0]} {order_result[1]} 개\n"
if order_result[2] > 0:
append_message = append_message + f"\t*{database['상품'][2][0]} {order_result[2]} 개\n"
if order_result[3] > 0:
append_message = append_message + f"\t*{database['상품'][3][0]} {order_result[3]} 개\n"
if order_result[4] > 0:
append_message = append_message + f"\t*{database['상품'][4][0]} {order_result[4]} 개\n"
if order_result[5] > 0:
append_message = append_message + f"\t*{database['상품'][5][0]} {order_result[5]} 개\n"
if order_result[6] > 0:
append_message = append_message + f"\t*{database['상품'][6][0]} {order_result[6]} 개\n"
if order_result[7] > 0:
append_message = append_message + f"\t*{database['상품'][7][0]} {order_result[7]} 개\n"
if order_result[8] > 0:
append_message = append_message + f"\t*{database['상품'][8][0]} {order_result[8]} 개\n"
if order_result[9] > 0:
append_message = append_message + f"\t*{database['상품'][9][0]} {order_result[9]} 개\n"
if order_result[10] > 0:
append_message = append_message + f"\t*{database['상품'][10][0]} {order_result[10]} 개\n"
if order_result[11] > 0:
append_message = append_message + f"\t*{database['상품'][11][0]} {order_result[11]} 개\n"
if is_possible_order:
# 주문 내역 출력
message = f"""[구매 물품 목록]
주문목록 :
{append_message} 총 가격 : {order_result['total_price']} 원
[주문 종료!]"""
print(message)

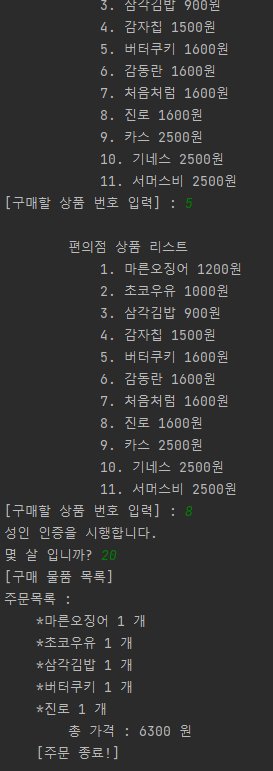
2번 문제와 비슷하게 현실 상황에 대해 구현을 하는 프로그래밍이었다. 마찬가지로 객체지향적으로 코딩을 하기 위해 'database'라는 하나의 딕셔너리 객체에서 모든 소스가 참조되도록 만들었다. 여기 내부만 수정하면 보여지는 상품의 이름, 가격, 성인인증 필요 여부까지 동일하게 작동할 수 있다. 또한 주문 순서와 상관 없게 주문 목록과 개수를 불러오기 때문에 디자인도 통일감을 챙겼다.
print('팀명: 동녘짱과 아이들')
print('[문제1] : 1, [문제2] : 2, [문제3] : 3')
print('팀명: 풀어보던가')
print('[문제1] : 4, [문제2] : 5, [문제3] : 6')
print('팀명: 똘똘이')
print('[문제1] : 7, [문제2] : 8, [문제3] : 9')
print('팀명: 월요일이조')
print('[문제1] : 10, [문제2] : 11, [문제3] : 12')
print('팀명: 경주마팸')
print('[문제1] : 13, [문제2] : 14, [문제3] : 15')
print('팀명: 김주김')
print('[문제1] : 16, [문제2] : 17, [문제3] : 18')
quest_select = int(input('어디 문제를 체험해보고 싶습니까?'))
개인적으로 학우님들이 올려준 문제를 잘 보존하기 위해 1개의 파일로 만들어 관리하려고 노력했다. 그러면서 단위 테스트도 부분적으로 잘 수행할 수 있게 로직을 짰다. 그래서 각 문제마다 quest_select의 여부를 확인하는 소스가 들어있는 이유다.
전체 소스코드가 1600줄 정도 되다보니, 점점 관리하기 힘들어졌다. 파이썬이 띄어쓰기로 구분하다보니, 중간에 소스 정렬이 의도대로 되지 않으면, 전체 들여쓰기가 초기화되어버리는 대참사가 일어났었다. 아무래도 """text""" 문법을 많이 쓰고, '+' 연산자를 붙여놓고 피연산자를 안넣어놓은 상태로 정렬해버리면 밑줄의 소스가 피연산자로 인식하려고 IDE에서 정렬를 해주다 보니 그런 것 같다.
그리하여 왜 모듈을 쓰고, 패키징을 하려하는지 다시 한 번 체감했으며, OOP에 위배되는 행동을 할수록 결국 본인과 팀이 점점 더 고통받게 될 것이라는 예견을 해본다. 처음 습관을 들일 때부터 잘 들이기 위해 앞으로도 계속 노력하겠다.
아직 문제가 더 남았으니.. 차차 정리해서 또 올리겠습니다.
감사합니다.
'광주인력개발원' 카테고리의 다른 글
<똘똘이> 팀이 내주신 문제 풀이 완료 [2023-04-19 학습일지] (0) | 2023.05.29 |
---|---|
<이것도 찾어보던가> 팀이 내주신 문제 풀이 완료 [2023-04-19 학습일지] (0) | 2023.05.29 |
문제 출제 및 학우님들 문제 풀어보기 [2023-04-17 학습일지] (0) | 2023.05.29 |
<개인프로젝트> 이세계 간호사 시뮬레이터 만들기 [2023-04-09 학습일지] (0) | 2023.05.29 |
로또 시뮬레이션 프로그램 만들기, 개발 계획서, 개발 완료 보고서, 소스코드 [2023-04-06 학습일지] (0) | 2023.05.29 |