PyQt5 공부하기 [2023-05-02 학습일지]
오늘부터 PyQt5 학습을 시작했다.
어떻게? "알아서 공부하기"
나는 이 방식이 좋다. 실제 업무를 하더라도 그렇게 다들 기술 스택을 쌓아가니까.
지금 당장엔 당황스럽고 어렵고 속도가 안나오더라도...
독립하고, 시니어가 되면 이제 알려주는 사람이 없다.
결국 언젠간 스스로 공부하고 터득할 줄 알아야하기 때문에.
이런 교육 방식은 의미있는 가르침이라 생각한다.
그리하여 오늘 스스로 공부한 내용은 무엇인가?
* PyQt5 내 모듈들의 종류와 간략한 요약
* PyQt5 의 클래스 상속 분석
* PyQt5 실제 타이핑 연습한 것
"""
* PyQt5 모듈
- Qt Core
: 그래픽과 관련되지 않은 핵심 클래스들
- Qt GUI
: OpenGL 을 포함한 기초적인 GUI 컴포넌트들
- Qt Multimedia
: 오디오, 비디오, 라디오, 카메라 기능들에 관한 클래스들
- Qt Multimedia Widgets
: 위젯 베이스의 멀티미디어 기능들
- Qt Network
: 네트워크 프로그래밍을 보다 쉽게 만들어주는 클래스들
- Qt QML
: Qt modeling language(QML) 과 JavaScript 언어에 관한 모듈
- Qt Quick
: 커스텀 인터페이스를 제공하는 동적 어플리케이션 제작에 필요한 선언적인 프레임 워크
- Qt Quick Controls
: 가벼운 QML 형식의 유저 인터페이스 제공 모듈, 데스크탑, 임베디드, 모바일에서 가볍게 적용 가능
- Qt Quick Dialogs
: Qt Quick apple에 필요한 System dialog를 만들어줌
- Qt Quick Layouts
: Quick 2 베이스를 기반한 레이아웃을 만들어줌
- Qt Quick Test
: QML 어플리케이션에 관한 유닛 테스트를 지원함. 자바스크립트로 쓰여짐
- Qt SQL
: 데이터베이스 통합 관한 클래스들
- Qt Test
: Qt 어플리케이션에 대한 유닛 테스트 관한 모듈
-Qt Widgets
: 넓은 범위의 GUI 클래스들 제공
"""
이것은 Qt4의 상속관계이다. (최근 이미지는 못찾음)
QWidget이 QtGUI에 소속되어 있는 모습이다.
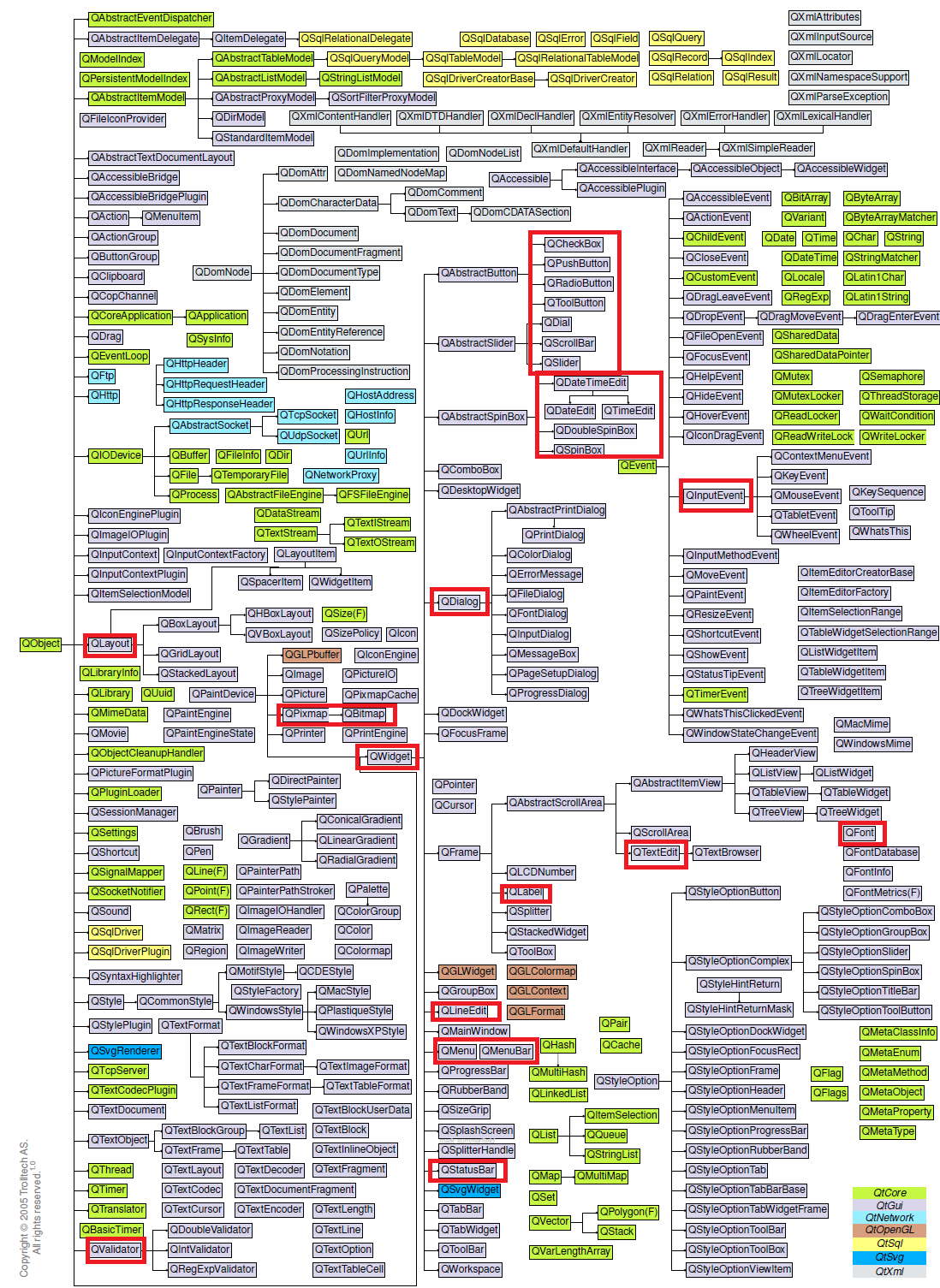
2일정도 PyQt를 공부하면서 봐온 함수들을 마킹해보았다.
import datetime
import sys
from PyQt5.QtWidgets import *
from PyQt5.QtCore import *
from PyQt5.QtGui import *
class Main(QDialog):
def __init__(self):
super().__init__()
self.config_ui()
def config_ui(self):
main_layout = QFormLayout()
# 이름 h-box-layout
name_layout = QHBoxLayout()
name_edit_line = QLineEdit()
name_layout.addWidget(name_edit_line)
# 생일 h-box-layout
birthday_layout = QHBoxLayout()
year_combo_box = QComboBox()
month_combo_box = QComboBox()
date_combo_box = QComboBox()
next_year = datetime.datetime.now().year + 1
year_combo_box.addItems([str(x) for x in range(1900, next_year)])
month_combo_box.addItems([str(x) for x in range(1, 13)])
date_combo_box.addItems([str(x) for x in range(1, 32)])
birthday_layout.addWidget(year_combo_box)
birthday_layout.addWidget(month_combo_box)
birthday_layout.addWidget(date_combo_box)
# 주소 h-box-layout
address_layout = QVBoxLayout()
address_box = QComboBox()
address_box.addItems([
"서울", "광주", "경기", "울산", "부산", "강원"
])
detail_address_edit_line = QLineEdit()
address_layout.addWidget(address_box)
address_layout.addWidget(detail_address_edit_line)
# 이메일 h-box-layout
email_layout = QHBoxLayout()
email_edit_line = QLineEdit()
email_company_line = QLineEdit()
email_company_combobox = QComboBox()
email_company_combobox.addItems([
"google.com", "naver.com", "daum.net"
])
email_layout.addWidget(email_edit_line)
email_layout.addWidget(QLabel("@"))
email_layout.addWidget(email_company_line)
email_layout.addWidget(email_company_combobox)
# 전화번호 h-box-layout
phone_number_layout = QHBoxLayout()
phone_number_front = QLineEdit()
phone_number_middle = QLineEdit()
phone_number_end = QLineEdit()
phone_number_layout.addWidget(phone_number_front)
phone_number_layout.addWidget(QLabel("-"))
phone_number_layout.addWidget(phone_number_middle)
phone_number_layout.addWidget(QLabel("-"))
phone_number_layout.addWidget(phone_number_end)
# 키 h-box-layout
height_layout = QHBoxLayout()
height_widget = QSpinBox()
height_widget.setValue(20)
height_layout.addWidget(height_widget)
# 개인정보 제공 h-box-layout
privacy_information_share_layout = QHBoxLayout()
privacy_info_share_agree_checkbox = QCheckBox()
privacy_info_share_agree_checkbox.setChecked(True)
privacy_information_share_layout.addWidget(privacy_info_share_agree_checkbox)
privacy_information_share_layout.addWidget(QLabel("동의"))
privacy_information_share_layout.addStretch()
# 플레인 텍스트 h-box-layout
self_introduction_layout = QHBoxLayout()
self_intro_text = QPlainTextEdit()
self_introduction_layout.addWidget(self_intro_text)
# Save or cancel button h-box-layout
save_cancel_layout = QHBoxLayout()
save_button = QPushButton()
save_button.setText("저장")
cancel_button = QPushButton()
cancel_button.setText("취소")
save_cancel_layout.addWidget(save_button)
save_cancel_layout.addWidget(cancel_button)
# add row on main layout
main_layout.addRow("이름: ", name_layout)
main_layout.addRow("생일: ", birthday_layout)
main_layout.addRow("주소: ", address_layout)
main_layout.addRow("E-mail: ", email_layout)
main_layout.addRow("전화번호: ", phone_number_layout)
main_layout.addRow("키: ", height_layout)
main_layout.addRow("개인정보제공 동의: ", privacy_information_share_layout)
main_layout.addRow("자기소개: ", self_introduction_layout)
main_layout.addRow("", save_cancel_layout)
# showing
self.setFont(QFont("맑은 고딕", 12))
self.setLayout(main_layout)
self.resize(300, 400)
self.show()
if __name__ == '__main__':
app = QApplication(sys.argv)
sample_window = Main()
app.exec()
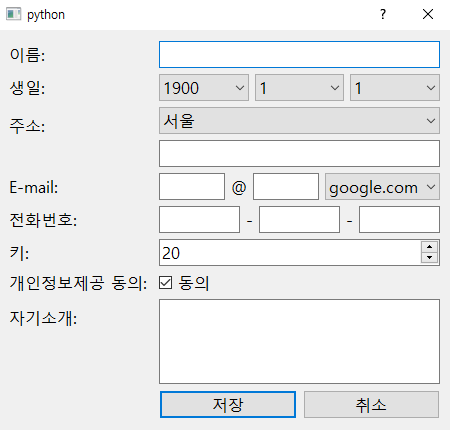
실행화면은 이렇다.
내일은 현재 Qt5 모듈에 대해 조금 더 분석해보고,
이것 저것 시도해볼 예정이다.
감사합니다.