광주인력개발원
for 반복문 사용하여 숫자 스무고개 만들기 [2023-04-21]
플광
2023. 5. 30. 08:50
"""
updown_game_230421_on_lecture
4/21 강의 내용
숫자 스무고개 만들기
"""
import random
import os
# TODO: 변수 선언
computer_select_num = None
user_select_num = None
min_num = 1
max_num = 99999
life_count = 20
remain_life = 1
intro_message = ["숫자 스무고개 게임입니다.", f"\n{min_num} ~ {max_num} 수 중에서 무엇을 내시겠습니까?\n",
'남은 기회는 ', ' 번 입니다.\n', '번호를 선택하세요. : ']
select_message = [' 를 입력하셨습니다.\n']
verified_message = ['잘못된 입력 입니다. 다시 입력 해주세요.\n']
verified_domain = [str(x) for x in range(min_num, max_num + 1)]
result_message = ['승리하였습니다.\n', '비겼습니다.\n', '패배하였습니다.\n',
'컴퓨터가 고른 수는 ', '였습니다.\n', '더 높습니다.\n', '더 낮습니다.\n']
statistic_message = ['총 전적은 ', '판입니다.', '승리 횟수 : ', '비긴 횟수 : ', '패배 횟수 : ', '승률 : ']
retry_ask_message = ['한번 더 하시겠습니까?\n', '[ 1. 계속 ] [ 2. 그만 ]\n', '번호를 선택하세요. : ']
exit_message = ['정상적으로 종료되었습니다.']
is_program_going = True
game_statistic = {
'total_play_count': 0,
'win_count': 0,
'lose_count': 0,
}
while is_program_going:
# TODO: 라이프 초기화
remain_life = life_count
os.system("cls") # 화면 지우기
computer_select_num = random.randrange(min_num, max_num)
print(intro_message[0], sep='', end='')
while remain_life > 0:
# ================내부 반복문 시작============================================ #
# TODO: 컴퓨터가 뽑은 수
game_result = 0 # 게임 결과에 따라 game_statistic 반영 예정
while True:
print(intro_message[1], sep='', end='')
print(intro_message[2], remain_life, intro_message[3], sep='', end='')
# TODO: 유저 입력
user_select_num = input()
# TODO: 유저 입력 검증
if user_select_num not in verified_domain:
print()
for m in verified_message:
print(m, sep='', end='\n')
else:
user_select_num = int(user_select_num)
print(user_select_num, end='')
print(select_message[0], end='')
break # 검증 완료
# TODO: 숫자 비교 로직
print(result_message[3], end='')
if user_select_num < computer_select_num:
print(result_message[5], end='')
elif user_select_num > computer_select_num:
print(result_message[6], end='')
else: # 같은 경우 : 승리 조건임
game_result = 1
# TODO: 만약 승리시엔 라이프 차감이 안되고 반복문을 탈출함
if game_result == 1:
break
# TODO: 라이프 차감
remain_life -= 1
# ================내부 반복문 종료============================================ #
# TODO: 반복문 탈출 시 remain life > 0이면 life 안에 정답을 맞춘 것이므로 승리 아니면 패배
if remain_life > 0:
game_result = 1
else:
game_result = 3
# TODO: 결과화면 출력
if game_result == 1: # 정답을 맞춘 경우
print(result_message[0], end='')
elif game_result == 3: # 정답을 못 맞춘 경우
print(result_message[2], end='')
print(result_message[3], computer_select_num, result_message[4], sep=' ', end='')
if computer_select_num == 1:
print(result_message[4])
elif computer_select_num == 3:
print(select_message[6])
# TODO: 승부 결과 통계 반영
game_statistic['total_play_count'] += 1
if game_result == 1: # 승리
game_statistic['win_count'] += 1
elif game_result == 3: # 패배
game_statistic['lose_count'] += 1
# TODO: 통계화면 출력
print(f"""{statistic_message[0]}{game_statistic['total_play_count']} {statistic_message[1]}
{statistic_message[2]}{game_statistic['win_count']}
{statistic_message[4]}{game_statistic['lose_count']}
{statistic_message[5]}{(game_statistic['win_count'] * 100) / game_statistic['total_play_count']:.2f} %
""")
# TODO: 다시 시작할지 물어보기
while True:
# TODO: 유저 입력
for m in retry_ask_message:
print(m, sep='', end='')
user_select_num = input()
# TODO: 유저 입력 검증
if user_select_num not in verified_domain[:2]: # 1, 2까지만 확인함
print()
for m in verified_message:
print(m, sep='', end='\n')
else:
user_select_num = int(user_select_num)
if user_select_num == 1: # 다시 시작하는 경우
pass
elif user_select_num == 2: # 그만하는 경우
is_program_going = False
break # 검증 완료
# 종료 메시지
for m in exit_message:
print(m, sep='', end='')
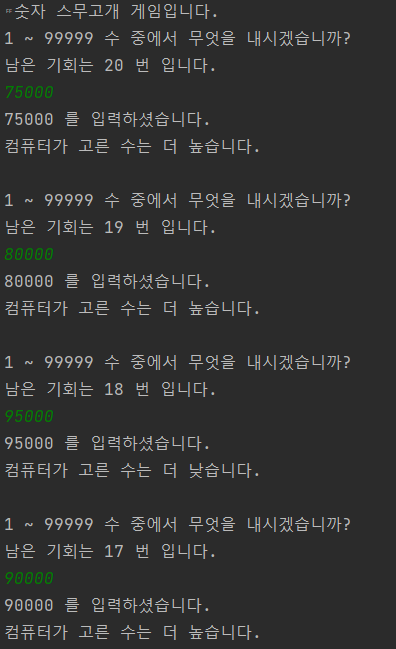
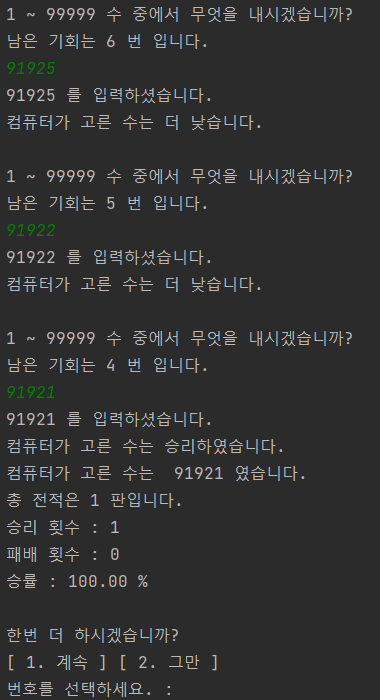
수업시간 과제로 숫자로 스무고개를 만들어보았다. 이전에 만든 가위바위보 게임의 틀에서 텍스트 메세지 일부와 변수 부분 조금 손을 봤고, 내부에 while이 하나 더 추가되어 remain_life 횟수만큼 반복하도록 로직을 수정했다. 그리하여, 통계 로직과 질문에 대해 검증하는 부분을 재활용할 수 있어 개발 시간을 단축시킬 수 있었다.
또한, 숫자 범위를 상단의 숫자만 조정해도, 전체 숫자 범위, 횟수를 조정하고, 알아서 텍스트까지 바꿔서 출력되기 때문에, 룰을 마음대로 조정할 수 있게 되었다. 아직은 부족한 객체지향적 프로그래밍이지만, 계속 시도를 해보도록 노력해야겠다.
감사합니다.