광주인력개발원
가위바위보 턴제 전투 게임 만들기, 개발 계획서, 소스 코드, 개발 완료 보고서 [2023-04-04 학습일지]
플광
2023. 5. 28. 20:33
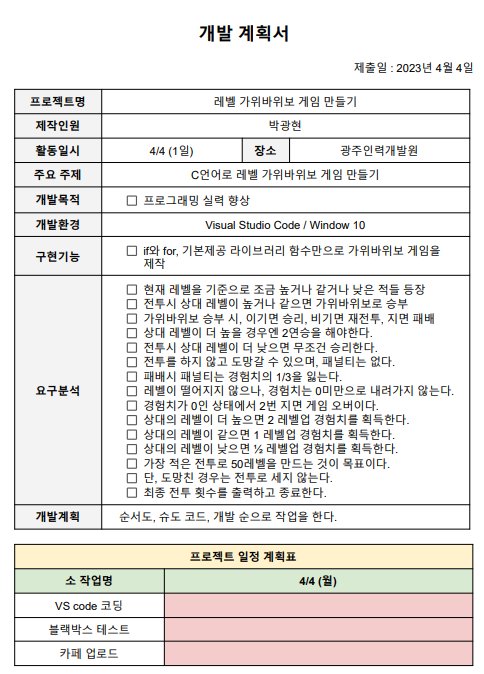
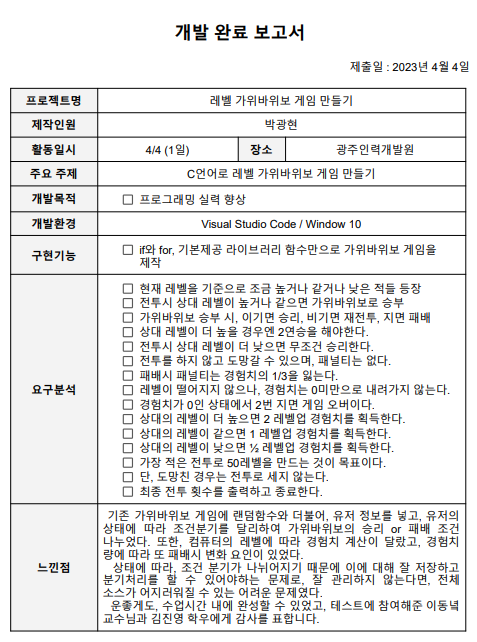
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <windows.h>
int main()
{
// 레벨 1 초기화
// 현재 레벨을 기준으로 조금 높거나 같거나 낮은 적들을 등장시킴
// 전투 시 상대 레벨이 더 높거나 같으면 가위바위보 승부
// 이기면 승리, 비기면 재전투, 지면 패배
// 단, 상대의 레벨이 더 높을 경우에는 가위바위보 승부를 2연승 해야함.
// 레벨이 같을 경우엔 단판 승부
// 레벨이 낮을 경우엔 전투를 하지 않고 승리로 간주함
// 전투를 하지 않고 도망갈 수 있으며, 패널티는 없음
// 패배시 경험치 1/3을 잃으나, 레벨이 떨어지지 않고, 0미만으로 내려가지 않음
// 단 경험치가 0인 상태에서 2번 지면, 게임 오버
//
// 상대의 레벨이 더 높으면 2 레벨업 경험치를 획득
// 상대의 레벨이 같으면 1 레벨업 경험치 획득
// 상대의 레벨이 낮으면 1/2 레벨업 경험치를 획득한다.
// 가장 적은 전투로 레벨을 50만드는 것이 목표
// 단 도망친 경우는 전투로 세지 않음.
// 최종 전투 횟수를 출력해주고 종료됨.
// 필요 변수
// 레벨업을 저장할 user_level, com_level,
// 경험치를 저장할 user_exp
// 경험치가 얼마가 되면 레벨업 할지, 얼마씩 증가시킬지 ? exp_max, exp_increase_unit
// 총 전투 횟수를 저장할 total_game_round
// 최종 레벨 저장할 level_limit_max
// 시작 레벨 저장할 level_limit_min
// 패배시 2연속인지 확인할 zero_lose_count
// 레벨 더 높은 상대일 때 2연승을 확인할 upper_battle_win_count
// 게임 오버 또는 승리시 재반복을 할지 여부 대해 물을 user_select_game_restart
// 다음 전투를 실행할지 여부에 대해 user_select_battle_start
int user_level, com_level;
int user_exp;
const int exp_max = 100;
const int exp_increase_unit = 50;
// 경험치 오르는 것이 1/2 씩 증가하므로 정수만 이용하면 2로 충분하나 많이 주는 느낌 주기 위해
// 100으로 설정함. 중간에 변하지 않아 지금 초기화, 증가량
int total_game_round;
int battle_result; // 배틀하고나면 초기값 0, 이기면 1, 비기면 2, 지면 3
const int level_limit_max = 50;
const int level_limit_min = 1;
const int have_to_win_count = 2; // 2번 이겨야 승리 조건
const int have_to_lose_count = 2; // 2번 이겨야 승리 조건
int zero_lose_count;
int upper_battle_win_count;
int user_select_game_restart = 1; // 게임가 시작할 수 있도록 1로 초기화
int user_select_battle_start = 1; // 전투가 시작할 수 있도록 1로 초기화
int user_select_fight_or_flight; // 전투에 돌입 시 도망칠지 여부 확인
int user_select_weapon; // 유저 가위, 바위, 보 선택을 저장
int com_select_weapon; // 컴퓨터 가위, 바위, 보 선택을 저장
srand(time(NULL));
while (user_select_game_restart == 1) // 최종 통계 화면 출력 후 재시작 여부 결정함
{
user_level = level_limit_min;
user_select_battle_start = 1;
user_exp = 2;
total_game_round = 0;
zero_lose_count = 0;
system("cls");
printf("게임 시작 \n");
while (user_select_battle_start == 1)
{
upper_battle_win_count = 0; // 매 라운드마다 초기화 해줄 변수
user_select_fight_or_flight = 0;
com_level = 0;
battle_result = 0;
if (user_level == 50) // 승리 조건
{
break;
}
if (zero_lose_count == have_to_lose_count) // 패배 조건
{
break;
}
if ((zero_lose_count < have_to_lose_count) && (zero_lose_count != 0))
{
printf("[[ 경고 : %d 번만 더 지면 게임 오버입니다. 집중!! ]]\n", (have_to_lose_count - zero_lose_count));
}
printf("\t\t\t\t\t\t\t\t[현재 레벨] %4d |[현재 경험치] %4d |[총 전투 경험] %4d|\n", user_level, user_exp, total_game_round);
if (total_game_round == 0)
{
printf("\t싸울까요? [1.전투] [2.나가기]\t\t ※ 한 번 나가면, 다시 돌아올 수 없습니다.\n");
}
if (total_game_round != 0)
{
printf("\t다시 싸울까요? [1.전투] [2.나가기]\t\t ※ 한 번 나가면, 다시 돌아올 수 없습니다.\n");
}
while (1)
{
scanf("%d", &user_select_battle_start);
getchar();
if (user_select_battle_start == 1) // 전투 진행 시
{
break;
}
if (user_select_battle_start == 2) // 전투 종료 시
{
break;
}
if (!((user_select_battle_start == 1) || (user_select_battle_start == 2))) // 잘못 입력한 경우
{
printf("잘못 입력하셨습니다. [1.전투] [2.나가기]\t\t ");
}
}
if (user_select_battle_start == 2) // 전투 종료 시, 외곽 while 종료하고 게임 통계 로직으로 넘어감
{
break;
}
// 컴퓨터 레벨 설정
do
{
com_level = user_level - (rand() % 4) + 2;
} while (com_level <= 0);
printf("lv.%2d 적이 등장했다.\n", com_level);
printf("싸우겠습니까? 도망치겠습니까? [1.싸우기] [2.도망가기]\t\t");
while (1)
{
scanf("%d", &user_select_fight_or_flight);
if (!(user_select_fight_or_flight == 1 || user_select_fight_or_flight == 2))
{ // 잘못 입력
printf("잘못 입력하셨습니다. [1.싸우기] [2.도망가기]\t\t ");
}
if ((user_select_fight_or_flight == 1 || user_select_fight_or_flight == 2))
{
break;
}
}
system("cls");
if (user_select_fight_or_flight == 1) // 싸우기 선택
{
battle_result = 0;
total_game_round++;
printf("전투다!!!\n");
do
{
if (user_level < com_level)
{
while (upper_battle_win_count < have_to_win_count)
{
user_select_weapon = 0;
if (upper_battle_win_count != 0)
{
printf("아직 %d 번 더 이겨야합니다.\n", (have_to_win_count - upper_battle_win_count));
}
printf("무기를 선택하세요. [1. 가위] [2. 바위] [3. 보]\t");
while (1)
{
scanf("%d", &user_select_weapon);
if (!(user_select_weapon == 1 || user_select_weapon == 2 || user_select_weapon == 3))
{ // 잘못 입력
printf("잘못 입력하셨습니다. [1. 가위] [2. 바위] [3. 보]\t ");
continue;
}
if ((user_select_weapon == 1 || user_select_weapon == 2 || user_select_weapon == 3))
{ // 옳은 입력
break;
}
}
com_select_weapon = rand() % 3 + 1;
if (user_select_weapon == 1) // 유저가 가위를 선택한 경우
{
if (com_select_weapon == 1)
{ // 컴퓨터가 가위를 선택한 경우
printf("무승부입니다.\n");
continue;
}
if (com_select_weapon == 2)
{ // 컴퓨터가 바위를 선택한 경우
printf("패배입니다.\n");
battle_result = 3;
break;
}
if (com_select_weapon == 3)
{ // 컴퓨터가 보를 선택한 경우
printf("승리입니다.\n");
upper_battle_win_count++;
if (upper_battle_win_count == have_to_win_count)
{
battle_result = 1;
break;
}
}
}
if (user_select_weapon == 2) // 유저가 바위를 선택한 경우
{
if (com_select_weapon == 1)
{ // 컴퓨터가 바위를 선택한 경우
printf("승리입니다.\n");
upper_battle_win_count++;
if (upper_battle_win_count == have_to_win_count)
{
battle_result = 1;
break;
}
}
if (com_select_weapon == 2)
{ // 컴퓨터가 가위를 선택한 경우
printf("무승부입니다.\n");
continue;
}
if (com_select_weapon == 3)
{ // 컴퓨터가 보를 선택한 경우
printf("패배입니다.\n");
battle_result = 3;
break;
}
}
if (user_select_weapon == 3) // 유저가 보를 선택한 경우
{
if (com_select_weapon == 1)
{ // 컴퓨터가 가위를 선택한 경우
printf("패배입니다.\n");
battle_result = 3;
break;
}
if (com_select_weapon == 2)
{ // 컴퓨터가 바위를 선택한 경우
printf("승리입니다.\n");
upper_battle_win_count++;
if (upper_battle_win_count == have_to_win_count)
{
battle_result = 1;
break;
}
}
if (com_select_weapon == 3)
{ // 컴퓨터가 보를 선택한 경우
printf("무승부입니다.\n");
continue;
}
}
}
} // user_level < com_level 끝
if (user_level == com_level)
{
// printf("자동 승리!\n");
// battle_result = 1;
user_select_weapon = 0;
printf("무기를 선택하세요. [1. 가위] [2. 바위] [3. 보]\t");
while (1)
{
scanf("%d", &user_select_weapon);
if (!(user_select_weapon == 1 || user_select_weapon == 2 || user_select_weapon == 3))
{ // 잘못 입력
printf("잘못 입력하셨습니다. [1. 가위] [2. 바위] [3. 보]\t ");
continue;
}
if ((user_select_weapon == 1 || user_select_weapon == 2 || user_select_weapon == 3))
{ // 옳은 입력
break;
}
}
com_select_weapon = rand() % 3 + 1;
if (user_select_weapon == 1) // 유저가 가위를 선택한 경우
{
if (com_select_weapon == 1)
{ // 컴퓨터가 가위를 선택한 경우
printf("무승부입니다.\n");
continue;
}
if (com_select_weapon == 2)
{ // 컴퓨터가 바위를 선택한 경우
printf("패배입니다.\n");
battle_result = 3;
break;
}
if (com_select_weapon == 3)
{ // 컴퓨터가 보를 선택한 경우
printf("승리입니다.\n");
battle_result = 1;
break;
}
}
if (user_select_weapon == 2) // 유저가 바위를 선택한 경우
{
if (com_select_weapon == 1)
{ // 컴퓨터가 바위를 선택한 경우
printf("승리입니다.\n");
battle_result = 1;
break;
}
if (com_select_weapon == 2)
{ // 컴퓨터가 가위를 선택한 경우
printf("무승부입니다.\n");
continue;
}
if (com_select_weapon == 3)
{ // 컴퓨터가 보를 선택한 경우
printf("패배입니다.\n");
battle_result = 3;
break;
}
}
if (user_select_weapon == 3) // 유저가 보를 선택한 경우
{
if (com_select_weapon == 1)
{ // 컴퓨터가 가위를 선택한 경우
printf("패배입니다.\n");
battle_result = 3;
break;
}
if (com_select_weapon == 2)
{ // 컴퓨터가 바위를 선택한 경우
printf("승리입니다.\n");
battle_result = 1;
break;
}
if (com_select_weapon == 3)
{ // 컴퓨터가 보를 선택한 경우
printf("무승부입니다.\n");
continue;
}
}
} // user_level == com_level 끝
if (user_level > com_level)
{
printf("컴퓨터 레벨이 낮아 자동 승리!\n");
battle_result = 1;
} // user_level > com_level 끝
} while (battle_result == 0 || battle_result == 2);
}
if (battle_result == 1) // 승리
{
zero_lose_count = 0; // 연패 종료
if (user_level < com_level)
{
user_exp += exp_increase_unit * 4;
}
if (user_level == com_level)
{
user_exp += exp_increase_unit * 2;
}
if (user_level > com_level)
{
user_exp += exp_increase_unit;
}
}
if (battle_result == 3) // 패배
{
if (user_exp == 0)
{
zero_lose_count++;
}
if (user_exp != 0)
{
user_exp /= 3;
}
}
if (exp_max <= user_exp) // 레벨업 조건
{
printf("오잉..? 레벨의 상태가");
for (int i = 0; i < 3; i++)
{
if (i < 2)
{
printf(".");
}
if (i == 2)
{
printf("?");
}
Sleep(1000);
}
system("cls");
printf(" ,--, ,---, \n,---.'| ,`--.' | \n| | : ,--, | : : \n: : | ,--.'| ,--, ,-.----. ' ' ; \n| ' : | | : ,'_ /| \\ / \\ | | | \n; ; ' .---. : : ' .--. | | : | : |' : ; \n' | |__ ,---. /. ./| ,---. | ' | ,'_ /| : . | | | .\\ :| | ' \n| | :.'| / \\ .-' . ' | / \\ ' | | | ' | | . . . : |: |' : | \n' : ;/ / |/___/ \: |/ / || | : | | ' | | | | | \\ :; | ; \n| | ./. ' / |. \\ ' . ' / |' : |__ : | | : ' ; | : . |`---'. | \n; : ; ' ; /| \\ \\ ' ; /|| | '.'| | ; ' | | ' : |`-' `--..`; \n| ,/ ' | / | \\ \\ ' | / |; : ; : | : ; ; | : : : .--,_ \n'---' | : | \\ \\ | : || , / ' : `--' \\| | : | |`. \n \\ \\ / '---\" \\ \\ / ---`-' : , .-./`---'.| `-- -`, ; \n `----' `----' `--`----' `---` '---`\" \n");
printf("\t축하합니다! 레벨업 조건이 되어 %d에서 ", user_level);
user_level += (user_exp / exp_max);
user_exp = user_exp % exp_max;
if (user_level >= level_limit_max)
{
user_level = level_limit_max;
}
printf("%d가 되었습니다.\n", user_level);
if (user_level == level_limit_max)
{
printf("경축!!! 만렙 %d 달성!! \n", level_limit_max);
}
}
if (user_select_fight_or_flight == 2) // 도망가기 선택
{
printf("성공적으로 도망갔다!!!\n");
}
}
// 승리 조건
if (user_level == 50)
{
printf(" __ __ ___ _ _ __ __ ___ _ _ _ \n o O O \\ \\ / / / _ \\ | | | |\\ \\ / /|_ _| | \\| | | | \n o \\ V / | (_) | | |_| | \\ \\/\\/ / | | | .` | |_| \n TS__[O] _|_|_ \\___/ \\___/ \\_/\\_/ |___| |_|\\_| _(_)_ \n {======|_| \"\"\" |_|\"\"\"\"\"|_|\"\"\"\"\"|_|\"\"\"\"\"|_|\"\"\"\"\"|_|\"\"\"\"\"|_| \"\"\" | \n./o--000'\"`-0-0-'\"`-0-0-'\"`-0-0-'\"`-0-0-'\"`-0-0-'\"`-0-0-'\"`-0-0- \n");
printf("승리.\n");
}
// 패배 조건
if (user_level < 50)
{
printf(" ____ ,----.. \n ,----.. ,---, ,' , `. ,---,. / / \\ ,---,.,-.----. \n / / \\ ' .' \\ ,-+-,.' _ | ,' .' | / . : ,---. ,' .' |\\ / \\ \n| : : / ; '. ,-+-. ; , ||,---.' | . / ;. \\ /__./|,---.' |; : \\ \n. | ;. / : : \\ ,--.'|' | ;|| | .' . ; / ` ; ,---.; ; || | .'| | .\\ : \n. ; /--` : | /\\ \\ | | ,', | ':: : |-, ; | ; \\ ; |/___/ \\ | |: : |-,. : |: | \n; | ; __ | : ' ;. : | | / | | ||: | ;/| | : | ; | '\\ ; \\ ' |: | ;/|| | \\ : \n| : |.' .'| | ;/ \\ \' | : | : |,| : .' . | ' ' ' : \\ \\ \\: || : .'| : . / \n. | '_.' :' : | \\ \\ ,'; . | ; |--' | | |-, ' ; \\; / | ; \\ ' .| | |-,; | | \\ \n' ; : \\ || | ' '--' | : | | , ' : ;/| \\ \\ ', / \\ \\ '' : ;/|| | ;\\ \\ \n' | '/ .'| : : | : ' |/ | | \\ ; : / \\ ` ;| | \\: ' | \\.' \n| : / | | ,' ; | |`-' | : .' \\ \\ .' : \\ || : .': : :-' \n \\ \\ .' `--'' | ;/ | | ,' `---` '---\" | | ,' | |.' \n `---` '---' `----' `----' `---' \n");
printf("게임 오버.\n");
}
// 전투 통계
printf("총 %d판 전투를 했습니다.\n", total_game_round);
printf("최종 레벨 : %d\n", user_level);
// 게임 재시작 여부
printf("다시 시작하시겠습니까? [1. 계속] [2. 종료] \t");
scanf("%d", &user_select_game_restart);
}
return 0;
}